c++ 三维 2线段最小距离 源代码
时间: 2024-02-07 15:04:14 浏览: 88
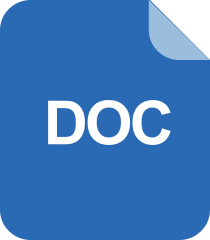
空间中2条线段的最短距离

以下是C++的三维两线段最小距离的源代码:
```c++
#include <bits/stdc++.h>
using namespace std;
double DotProduct(double x1, double y1, double z1, double x2, double y2, double z2) {
return x1 * x2 + y1 * y2 + z1 * z2;
}
double CrossProduct(double x1, double y1, double z1, double x2, double y2, double z2, double& x, double& y, double& z) {
x = y1 * z2 - y2 * z1;
y = z1 * x2 - z2 * x1;
z = x1 * y2 - x2 * y1;
return sqrt(x * x + y * y + z * z);
}
double Distance(double x1, double y1, double z1, double x2, double y2, double z2) {
return sqrt((x1 - x2) * (x1 - x2) + (y1 - y2) * (y1 - y2) + (z1 - z2) * (z1 - z2));
}
double SegmentAndSegment(double x1, double y1, double z1, double x2, double y2, double z2, double x3, double y3, double z3, double x4, double y4, double z4) {
double x, y, z;
double a1 = x2 - x1;
double b1 = y2 - y1;
double c1 = z2 - z1;
double a2 = x4 - x3;
double b2 = y4 - y3;
double c2 = z4 - z3;
double a3 = x1 - x3;
double b3 = y1 - y3;
double c3 = z1 - z3;
double d1 = DotProduct(a1, b1, c1, a2, b2, c2);
double d2 = DotProduct(a1, b1, c1, a1, b1, c1);
double d3 = DotProduct(a2, b2, c2, a2, b2, c2);
double d4 = DotProduct(a1, b1, c1, a3, b3, c3);
double d5 = DotProduct(a2, b2, c2, a3, b3, c3);
double d = d2 * d3 - d1 * d1;
double t1, t2;
if (d == 0) {
t1 = 0;
t2 = (d1 > d3 ? d1 / d2 : d3 / d2);
} else {
t1 = (d1 * d5 - d2 * d4) / d;
t2 = (d1 * t1 + d5) / d3;
}
t1 = max(0.0, min(t1, 1.0));
t2 = max(0.0, min(t2, 1.0));
double u1 = x1 + t1 * a1;
double v1 = y1 + t1 * b1;
double w1 = z1 + t1 * c1;
double u2 = x3 + t2 * a2;
double v2 = y3 + t2 * b2;
double w2 = z3 + t2 * c2;
return Distance(u1, v1, w1, u2, v2, w2);
}
int main() {
double x1, y1, z1, x2, y2, z2, x3, y3, z3, x4, y4, z4;
cin >> x1 >> y1 >> z1 >> x2 >> y2 >> z2 >> x3 >> y3 >> z3 >> x4 >> y4 >> z4;
cout << SegmentAndSegment(x1, y1, z1, x2, y2, z2, x3, y3, z3, x4, y4, z4) << endl;
return 0;
}
```
其中,`DotProduct`函数计算向量的点积,`CrossProduct`函数计算向量的叉积,`Distance`函数计算两点之间的距离,`SegmentAndSegment`函数计算两条线段之间的最小距离。
阅读全文
相关推荐
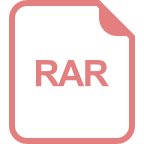

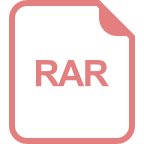
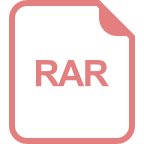
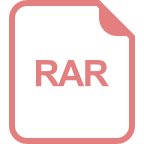
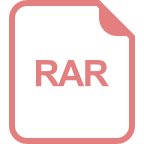
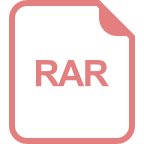
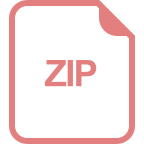
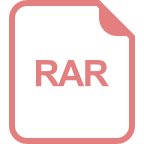
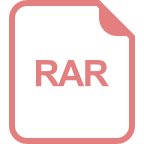
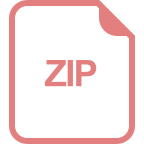
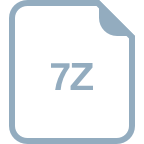
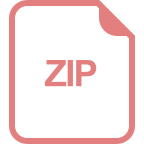
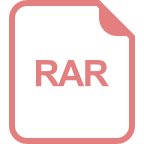
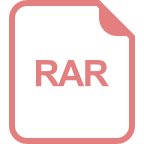
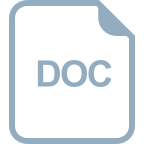