pta6-2 字符串的删除
时间: 2024-06-02 09:08:26 浏览: 13
题目描述:
给定一个字符串 $s$ 和一个字符集合 $t$,然后从字符串 $s$ 中删除所有包含字符集合 $t$ 中的字符的子串。你需要返回最终得到的字符串。
注意:
- 字符集合 $t$ 中的字符需要被删除,不能保留;
- 子串需要连续,即不能删除一个字符之后再删除它后面的字符。
示例 1:
输入: s = "leetcode", t = "e"
输出: "lcod"
解释: 在字符串中,包含字符集合 t 中的字符 e 的子串有 "e"、"ee" 和 "eet",所以将它们都删除,得到字符串 "lcod"。
示例 2:
输入: s = "abcdefg", t = "ab"
输出: "cdefg"
解释: 在字符串中,包含字符集合 t 中的字符 a 和 b 的子串有 "ab",所以将它们都删除,得到字符串 "cdefg"。
提示:
- 1 <= s.length <= $10^4$
- 1 <= t.length <= $100$
- s 中可能包含重复的字符,且 t 中的字符互不相同。
算法:
遍历字符串 $s$,每次遇到字符集合 $t$ 中的字符,就从这个位置开始删除不包含 $t$ 中字符的最长子串。这个过程可以使用双指针实现。
代码实现:
相关问题
c语言pta7-1删除数字字符
以下是C语言实现删除数字字符的代码:
```c
#include <stdio.h>
#include <string.h>
int main()
{
char str[10000];
char new_str[10000];
int i, j = 0;
gets(str);
for (i = 0; i < strlen(str); i++) {
if (str[i] < '0' || str[i] > '9') {
new_str[j] = str[i];
j++;
}
}
new_str[j] = '\0';
printf("%s", new_str);
return 0;
}
```
该代码通过遍历输入的字符串,将非数字字符存入新的字符串中,最后输出新的字符串即可实现删除数字字符的功能。
c语言删除字符串中的子串pta
根据引用[1]和引用,本题需要实现删除字符串中的子串pta。具体实现步骤如下:
1.定义一个函数,输入为原始字符串和需要删除的子串,输出为删除子串后的字符串。
2.遍历原始字符串,如果当前字符与子串的第一个字符相同,则判断后续字符是否与子串相同,如果相同则删除该子串。
3.将删除子串后的字符串返回。
以下是C语言实现代码:
```c
#include <stdio.h>
#include <string.h>
char* deleteSubStr(char* str, char* subStr) {
int len1 = strlen(str);
int len2 = strlen(subStr);
int i, j, k;
for (i = 0; i < len1; i++) {
if (str[i] == subStr[0]) {
for (j = 1, k = i + 1; j < len2 && k < len1; j++, k++) {
if (str[k] != subStr[j]) {
break;
}
}
if (j == len2) {
memmove(&str[i], &str[k], len1 - k + 1);
i--;
len1 = strlen(str);
}
}
}
return str;
}
int main() {
char str[1001], subStr[] = "pta";
fgets(str, 1001, stdin);
str[strlen(str) - 1] = '\0';
printf("%s", deleteSubStr(str, subStr));
return 0;
}
```
相关推荐







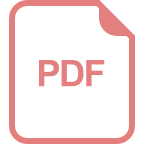
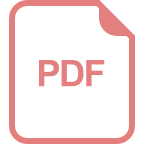
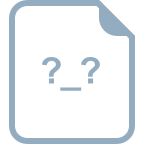