java实现磁盘调度算法的模拟实现及对比
时间: 2023-12-18 11:05:45 浏览: 82
磁盘调度算法是操作系统中用于管理磁盘的一种算法,它的主要目的是优化磁盘的读写效率,提高磁盘的利用率。常见的磁盘调度算法有:FCFS(先来先服务)、SSTF(最短寻道时间优先)、SCAN(扫描算法)、C-SCAN(循环扫描算法)等。下面是 Java 实现磁盘调度算法的模拟实现及对比。
1. FCFS(先来先服务)
FCFS 算法是最简单的磁盘调度算法,它按照请求的先后顺序进行磁盘访问,即先请求的先被服务。Java 代码如下:
```java
public class FCFS {
public static void main(String[] args) {
int[] requests = {40, 10, 35, 80, 25};
int head = 50;
int total = 0;
for (int i = 0; i < requests.length; i++) {
int distance = Math.abs(requests[i] - head);
total += distance;
head = requests[i];
}
System.out.println("Total Seek Time: " + total);
}
}
```
2. SSTF(最短寻道时间优先)
SSTF 算法是按照当前磁头位置与请求队列中下一个请求的磁道距离最近,优先服务距离最短的请求。Java 代码如下:
```java
public class SSTF {
public static void main(String[] args) {
int[] requests = {40, 10, 35, 80, 25};
int head = 50;
int total = 0;
while (requests.length > 0) {
int minDistance = Integer.MAX_VALUE;
int minIndex = -1;
for (int i = 0; i < requests.length; i++) {
int distance = Math.abs(requests[i] - head);
if (distance < minDistance) {
minDistance = distance;
minIndex = i;
}
}
total += minDistance;
head = requests[minIndex];
int[] newRequests = new int[requests.length - 1];
int j = 0;
for (int i = 0; i < requests.length; i++) {
if (i != minIndex) {
newRequests[j++] = requests[i];
}
}
requests = newRequests;
}
System.out.println("Total Seek Time: " + total);
}
}
```
3. SCAN(扫描算法)
SCAN 算法是按照磁头当前位置对请求进行扫描,服务请求队列中当前磁头位置下方的请求,直到最底端,然后改变磁头移动方向,服务上方的请求,直到最顶端,循环执行。Java 代码如下:
```java
public class SCAN {
public static void main(String[] args) {
int[] requests = {40, 10, 35, 80, 25};
int head = 50;
int total = 0;
int direction = 1; // 1: move to right, -1: move to left
Arrays.sort(requests);
int index = Arrays.binarySearch(requests, head);
if (index < 0) {
index = -(index + 1);
}
while (index < requests.length && index >= 0) {
int distance = Math.abs(requests[index] - head);
total += distance;
head = requests[index];
if (index == requests.length - 1) {
direction = -1;
}
index += direction;
}
total += head;
head = 0;
while (index < requests.length && index >= 0) {
int distance = Math.abs(requests[index] - head);
total += distance;
head = requests[index];
index += direction;
}
System.out.println("Total Seek Time: " + total);
}
}
```
4. C-SCAN(循环扫描算法)
C-SCAN 算法是类似 SCAN 算法,不同之处在于磁头到达磁盘底端时,不返回磁盘顶端,而是直接返回磁盘底端,形成一个循环执行的过程。Java 代码如下:
```java
public class CSCAN {
public static void main(String[] args) {
int[] requests = {40, 10, 35, 80, 25};
int head = 50;
int total = 0;
int direction = 1; // 1: move to right, -1: move to left
Arrays.sort(requests);
int index = Arrays.binarySearch(requests, head);
if (index < 0) {
index = -(index + 1);
}
while (index < requests.length && index >= 0) {
int distance = Math.abs(requests[index] - head);
total += distance;
head = requests[index];
if (index == requests.length - 1) {
direction = -1;
total += (requests[requests.length - 1] - requests[0]);
head = 0;
index = Arrays.binarySearch(requests, head);
if (index < 0) {
index = -(index + 1);
}
} else {
index += direction;
}
}
System.out.println("Total Seek Time: " + total);
}
}
```
以上就是 Java 实现磁盘调度算法的模拟实现及对比。根据不同的磁盘访问情况,选择不同的磁盘调度算法,可以有效提高磁盘的读写效率,提高系统的性能。
阅读全文
相关推荐
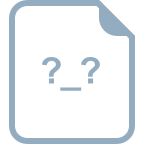

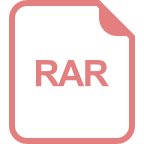
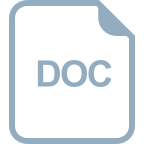
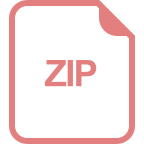
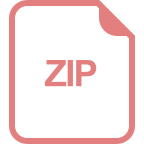
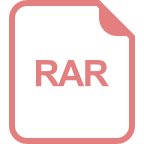
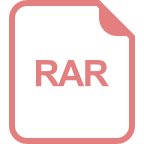
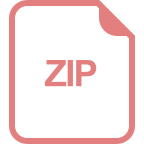
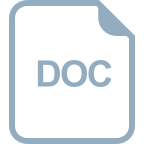
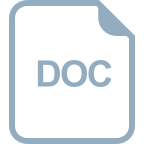
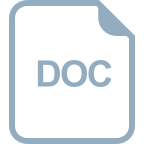



