用python将多个字符串按ASCII 码从小到大排序
时间: 2024-05-11 13:17:24 浏览: 139
可以使用Python内置的`sorted`函数来实现字符串按ASCII码从小到大排序。具体实现方法如下:
```python
strings = ['apple', 'zebra', 'banana', 'orange']
sorted_strings = sorted(strings)
print(sorted_strings)
```
输出结果:
```
['apple', 'banana', 'orange', 'zebra']
```
在这个例子中,我们定义了一个字符串列表`strings`,并使用`sorted`函数将其按ASCII码从小到大排序,存储在变量`sorted_strings`中。最后,我们输出排好序的字符串列表`sorted_strings`。
相关问题
用起泡法将字符串按ASCII码值从小到大排列
好的,下面是用起泡法实现字符串按ASCII码值从小到大排列的 Python 代码:
```python
def bubble_sort_str(s):
n = len(s)
# 转换为字符数组
arr = list(s)
# 起泡排序
for i in range(n):
for j in range(n-i-1):
if ord(arr[j]) > ord(arr[j+1]):
arr[j], arr[j+1] = arr[j+1], arr[j]
# 转换为字符串
res = ''.join(arr)
return res
```
这个函数接受一个字符串作为参数,返回按ASCII码值从小到大排列后的字符串。具体实现是将字符串转换为字符数组,使用起泡排序算法进行排序,然后再将字符数组转换为字符串。其中,`ord()` 函数可以将字符转换为对应的 ASCII 码值。
使用起泡法和选择法将字符串按ASCII码值从小到大排列
好的,让我来为你解答这个问题。
首先,让我们来看看使用起泡法将字符串按ASCII码值从小到大排列的过程:
```python
def bubble_sort(s):
n = len(s)
for i in range(n):
for j in range(n-i-1):
if ord(s[j]) > ord(s[j+1]):
s[j], s[j+1] = s[j+1], s[j]
return s
s = "hello, world!"
s = list(s)
print(bubble_sort(s))
```
使用选择法将字符串按ASCII码值从小到大排列的过程如下:
```python
def selection_sort(s):
n = len(s)
for i in range(n):
min_idx = i
for j in range(i+1, n):
if ord(s[j]) < ord(s[min_idx]):
min_idx = j
s[i], s[min_idx] = s[min_idx], s[i]
return s
s = "hello, world!"
s = list(s)
print(selection_sort(s))
```
以上就是使用起泡法和选择法将字符串按ASCII码值从小到大排列的过程,希望对你有所帮助!
阅读全文
相关推荐
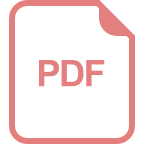
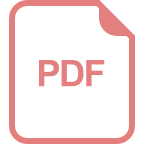













