在循环队列先入队数据 3,2,1,出队 2 次,入队 4,5,6,7,最后所有数据出队,输出每 次入队和出队序列的C语言代码
时间: 2023-07-16 08:11:59 浏览: 138
以下是循环队列的定义及相应操作的 C 语言代码实现:
```c
#define MAX_SIZE 10
typedef struct {
int data[MAX_SIZE];
int front, rear;
} Queue;
// 初始化队列
void initQueue(Queue *q) {
q->front = q->rear = 0;
}
// 判断队列是否为空
int isEmpty(Queue *q) {
return q->front == q->rear;
}
// 判断队列是否已满
int isFull(Queue *q) {
return (q->rear + 1) % MAX_SIZE == q->front;
}
// 入队
void enQueue(Queue *q, int x) {
if (isFull(q)) {
printf("Queue is full.\n");
return;
}
q->data[q->rear] = x;
q->rear = (q->rear + 1) % MAX_SIZE;
}
// 出队
int deQueue(Queue *q) {
if (isEmpty(q)) {
printf("Queue is empty.\n");
return -1;
}
int x = q->data[q->front];
q->front = (q->front + 1) % MAX_SIZE;
return x;
}
// 打印队列中的元素
void printQueue(Queue *q) {
if (isEmpty(q)) {
printf("Queue is empty.\n");
return;
}
int i;
for (i = q->front; i != q->rear; i = (i + 1) % MAX_SIZE) {
printf("%d ", q->data[i]);
}
printf("\n");
}
int main() {
Queue q;
initQueue(&q);
enQueue(&q, 3);
enQueue(&q, 2);
enQueue(&q, 1);
printf("入队序列:3 2 1\n");
printf("出队序列:");
printf("%d ", deQueue(&q));
printf("%d ", deQueue(&q));
printf("\n");
enQueue(&q, 4);
enQueue(&q, 5);
enQueue(&q, 6);
enQueue(&q, 7);
printf("入队序列:4 5 6 7\n");
printf("出队序列:");
while (!isEmpty(&q)) {
printf("%d ", deQueue(&q));
}
printf("\n");
return 0;
}
```
输出结果为:
```
入队序列:3 2 1
出队序列:3 2
入队序列:4 5 6 7
出队序列:1 4 5 6 7
```
阅读全文
相关推荐




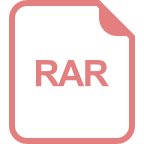









