用C++编写一个商店货栈
时间: 2024-10-10 08:07:10 浏览: 18
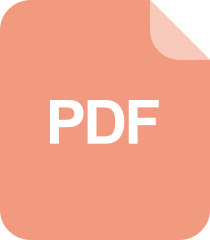
基于二层信用策略的两货栈库存模型 (2010年)
在C++中,我们可以创建一个基本的商店货栈(Stack)数据结构,通常会使用数组或动态存储容器如`std::stack`来实现。下面是一个简单的例子,演示如何使用数组实现一个名为`ShoppingCartStack`的货栈:
```cpp
#include <iostream>
#include <vector>
class ShoppingCartStack {
private:
std::vector<int> items;
int top; // 栈顶元素的索引
public:
// 初始化空栈
ShoppingCartStack() : top(-1) {}
// 判断栈是否为空
bool isEmpty() const { return top == -1; }
// 入栈操作
void push(int item) {
if (top >= items.size() - 1) {
items.resize(items.size() * 2); // 扩容栈
}
items[++top] = item;
}
// 出栈操作
int pop() {
if (isEmpty()) {
throw std::runtime_error("Stack is empty");
}
return items[top--];
}
// 查看栈顶元素
int peek() const {
if (isEmpty()) {
throw std::runtime_error("Stack is empty");
}
return items[top];
}
// 返回栈的大小
size_t size() const { return top + 1; }
};
int main() {
ShoppingCartStack cart;
cart.push(1);
cart.push(2);
cart.push(3);
std::cout << "Top item: " << cart.peek() << "\n";
std::cout << "Size: " << cart.size() << "\n";
while (!cart.isEmpty()) {
std::cout << "Popped: " << cart.pop() << "\n";
}
return 0;
}
```
在这个示例中,我们创建了一个`ShoppingCartStack`类,它有基本的入栈(push)、出栈(pop)和查看栈顶(peek)功能。注意,这个例子使用了数组作为底层数据结构,当栈满时会自动扩容。
阅读全文
相关推荐
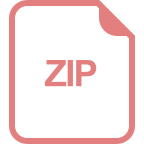
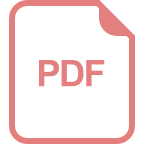



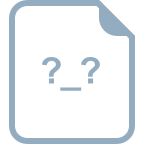
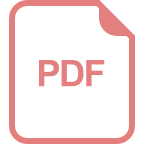
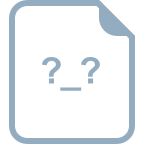
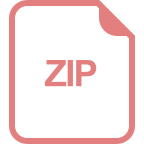
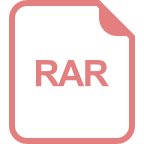
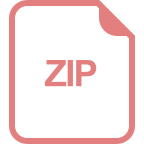
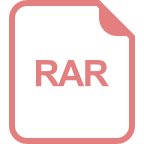
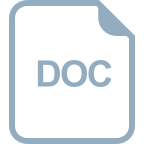



