用java写贪吃蛇游戏,用上下左右键盘控制
时间: 2023-05-25 15:01:55 浏览: 36
A:好的,下面是一个简单的Java贪吃蛇游戏的代码实现,使用上下左右键盘控制蛇的移动方向。
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class SnakeGame extends JFrame implements KeyListener {
private int x = 50, y = 50; // 初始蛇头位置
private int step = 10; // 每步移动距离
private int direction = 0; // 初始移动方向为右
private int[] snakeX = new int[500]; // 蛇的X坐标数组
private int[] snakeY = new int[500]; // 蛇的Y坐标数组
private int foodX, foodY; // 食物位置
private int score = 0; // 得分
private boolean gameOver = false; // 游戏结束标志
public SnakeGame() {
setTitle("贪吃蛇游戏");
setSize(500, 500);
setLocation(100, 100);
setVisible(true);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
addKeyListener(this);
// 初始化蛇的位置
snakeX[0] = x;
snakeY[0] = y;
// 随机产生食物位置
foodX = (int) (Math.random() * 480);
foodY = (int) (Math.random() * 480);
}
public void paint(Graphics g) {
super.paint(g);
if (!gameOver) {
// 画蛇头
g.setColor(Color.RED);
g.fillOval(x, y, 10, 10);
// 画蛇身
g.setColor(Color.BLACK);
for (int i = 1; i < score; i++) {
g.fillOval(snakeX[i], snakeY[i], 10, 10);
}
// 画食物
g.setColor(Color.GREEN);
g.fillOval(foodX, foodY, 10, 10);
// 显示分数
g.setColor(Color.BLUE);
g.drawString("得分:" + score, 10, 470);
} else {
// 游戏结束,显示分数
g.setColor(Color.BLUE);
g.drawString("游戏结束,得分:" + score, 200, 250);
}
}
public void keyPressed(KeyEvent e) {
int keyCode = e.getKeyCode();
if (keyCode == KeyEvent.VK_UP) { // 上
if (direction != 2) {
direction = 1;
}
} else if (keyCode == KeyEvent.VK_DOWN) { // 下
if (direction != 1) {
direction = 2;
}
} else if (keyCode == KeyEvent.VK_LEFT) { // 左
if (direction != 4) {
direction = 3;
}
} else if (keyCode == KeyEvent.VK_RIGHT) { // 右
if (direction != 3) {
direction = 4;
}
}
}
public void keyReleased(KeyEvent arg0) {
}
public void keyTyped(KeyEvent arg0) {
}
public void move() {
// 移动蛇
for (int i = score; i > 0; i--) {
snakeX[i] = snakeX[i - 1];
snakeY[i] = snakeY[i - 1];
}
if (direction == 1) { // 上
y -= step;
} else if (direction == 2) { // 下
y += step;
} else if (direction == 3) { // 左
x -= step;
} else if (direction == 4) { // 右
x += step;
}
// 判断是否吃到食物
if (x == foodX && y == foodY) {
score++;
// 重新随机产生食物位置
foodX = (int) (Math.random() * 480);
foodY = (int) (Math.random() * 480);
}
// 判断是否撞墙或撞到自己的身体
for (int i = 1; i < score; i++) {
if (x == snakeX[i] && y == snakeY[i]) {
gameOver = true;
}
}
if (x < 0 || x > 490 || y < 0 || y > 490) {
gameOver = true;
}
repaint(); // 重新绘制界面
}
public static void main(String[] args) {
SnakeGame game = new SnakeGame();
while (!game.gameOver) {
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
game.move();
}
}
}
```
相关推荐
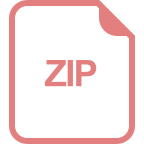
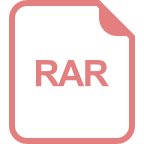
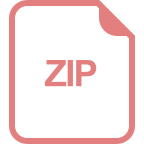
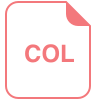
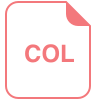
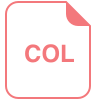
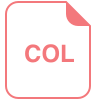
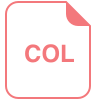









