你可以用java写一个贪吃蛇小游戏吗,要求用“W”“S”“A”“D”控制上下左右移动
时间: 2023-09-02 19:07:35 浏览: 82
是的,我可以给您提供Java写的贪吃蛇小游戏示例代码,以下是一个简单的实现,使用W、S、A、D控制上下左右移动:
```java
import java.awt.Color;
import java.awt.Font;
import java.awt.Graphics;
import java.awt.Point;
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
import java.util.ArrayList;
import java.util.List;
import java.util.Random;
import javax.swing.JFrame;
import javax.swing.JPanel;
/**
* Java贪吃蛇小游戏示例代码
*/
public class SnakeGame extends JPanel implements KeyListener {
private static final long serialVersionUID = 1L;
private final int WIDTH = 800; // 游戏界面宽度
private final int HEIGHT = 600; // 游戏界面高度
private final int BLOCK_SIZE = 20; // 单元格大小
private List<Point> snake; // 蛇的集合
private Point food; // 食物坐标
private int direction; // 蛇的移动方向(0-上,1-下,2-左,3-右)
private boolean isOver; // 是否结束游戏
private int score; // 得分
public SnakeGame() {
init(); // 初始化游戏
JFrame frame = new JFrame("Java贪吃蛇小游戏"); // 创建游戏窗口
frame.setSize(WIDTH, HEIGHT);
frame.setLocationRelativeTo(null);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setResizable(false);
frame.add(this);
frame.setVisible(true);
this.addKeyListener(this); // 添加键盘监听器
this.setFocusable(true); // 设置焦点(用于监听键盘事件)
}
/**
* 初始化游戏
*/
private void init() {
snake = new ArrayList<>();
snake.add(new Point(10, 10));
snake.add(new Point(10, 11));
snake.add(new Point(10, 12));
generateFood();
direction = 0;
isOver = false;
score = 0;
}
/**
* 生成食物
*/
private void generateFood() {
Random random = new Random();
int x = random.nextInt(WIDTH / BLOCK_SIZE);
int y = random.nextInt(HEIGHT / BLOCK_SIZE);
food = new Point(x, y);
}
/**
* 绘制游戏界面
*/
public void paint(Graphics g) {
super.paint(g);
g.setColor(Color.BLACK);
g.fillRect(0, 0, WIDTH, HEIGHT);
g.setColor(Color.WHITE);
g.setFont(new Font("宋体", Font.BOLD, 20));
g.drawString("得分:" + score, 20, 30);
if (isOver) {
g.setFont(new Font("宋体", Font.BOLD, 60));
g.drawString("游戏结束", 250, 300);
return;
}
// 绘制蛇
g.setColor(Color.GREEN);
for (Point p : snake) {
g.fillRect(p.x * BLOCK_SIZE, p.y * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE);
}
// 绘制食物
g.setColor(Color.RED);
g.fillRect(food.x * BLOCK_SIZE, food.y * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE);
}
/**
* 检查是否碰撞到边界或自己的身体
*/
private boolean checkCollision() {
Point head = snake.get(0);
if (head.x < 0 || head.x >= WIDTH / BLOCK_SIZE || head.y < 0 || head.y >= HEIGHT / BLOCK_SIZE) {
return true; // 碰撞到边界
}
for (int i = 1; i < snake.size(); i++) {
Point p = snake.get(i);
if (p.x == head.x && p.y == head.y) {
return true; // 碰撞到自己的身体
}
}
return false;
}
/**
* 处理键盘事件
*/
public void keyPressed(KeyEvent e) {
int key = e.getKeyCode();
if (key == KeyEvent.VK_W && direction != 1) {
direction = 0; // 上
} else if (key == KeyEvent.VK_S && direction != 0) {
direction = 1; // 下
} else if (key == KeyEvent.VK_A && direction != 3) {
direction = 2; // 左
} else if (key == KeyEvent.VK_D && direction != 2) {
direction = 3; // 右
}
}
public void keyReleased(KeyEvent e) {
}
public void keyTyped(KeyEvent e) {
}
/**
* 游戏主循环
*/
public void run() throws InterruptedException {
while (!isOver) {
// 移动蛇的身体
for (int i = snake.size() - 1; i > 0; i--) {
Point p1 = snake.get(i - 1);
Point p2 = snake.get(i);
p2.x = p1.x;
p2.y = p1.y;
}
// 移动蛇的头部
Point head = snake.get(0);
if (direction == 0) {
head.y--;
} else if (direction == 1) {
head.y++;
} else if (direction == 2) {
head.x--;
} else if (direction == 3) {
head.x++;
}
// 检查是否吃到食物
if (head.x == food.x && head.y == food.y) {
snake.add(new Point(food.x, food.y));
generateFood();
score += 10;
}
// 检查是否碰撞到边界或自己的身体
if (checkCollision()) {
isOver = true;
}
// 重新绘制游戏界面
this.repaint();
// 等待一段时间,控制蛇的移动速度
Thread.sleep(100);
}
}
public static void main(String[] args) throws InterruptedException {
SnakeGame game = new SnakeGame();
game.run();
}
}
```
希望能对您有所帮助!
阅读全文
相关推荐



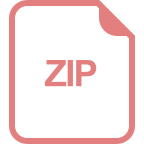
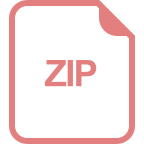
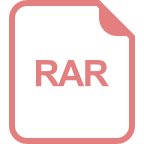
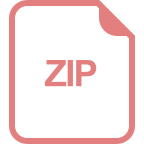
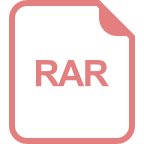
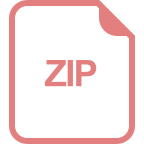