QT用多线程实现局域网IP端口扫描
时间: 2023-07-17 20:07:56 浏览: 311
在QT中,可以使用多线程来实现局域网IP端口扫描。下面是一个简单的示例代码:
```cpp
#include <QCoreApplication>
#include <QThread>
#include <QDebug>
#include <QTcpSocket>
class PortScanner : public QObject
{
Q_OBJECT
public:
explicit PortScanner(QObject *parent = nullptr) : QObject(parent) {}
public slots:
void scanPort(const QString &ipAddress, int port)
{
QTcpSocket socket;
socket.connectToHost(ipAddress, port);
if (socket.waitForConnected(1000)) {
qDebug() << "Port" << port << "on" << ipAddress << "is open.";
} else {
qDebug() << "Port" << port << "on" << ipAddress << "is closed.";
}
socket.disconnectFromHost();
}
};
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
PortScanner scanner;
QString ipAddress = "192.168.0."; // 局域网内的IP地址前缀
int startPort = 1; // 扫描起始端口号
int endPort = 65535; // 扫描结束端口号
for (int port = startPort; port <= endPort; ++port) {
for (int i = 1; i <= 255; ++i) {
QString fullIpAddress = ipAddress + QString::number(i);
QThread *thread = new QThread;
QObject::connect(thread, &QThread::started, [&]() {
scanner.scanPort(fullIpAddress, port);
thread->quit();
});
scanner.moveToThread(thread);
thread->start();
}
}
return a.exec();
}
#include "main.moc"
```
上述代码创建了一个`PortScanner`类,其中的`scanPort()`函数用于扫描指定IP地址的指定端口是否开放。主函数中通过循环遍历局域网内的IP地址和端口号,并为每个IP地址和端口号创建一个新的线程,然后在新线程中执行端口扫描操作。
在`scanPort()`函数中,使用`QTcpSocket`连接到指定的IP地址和端口,并通过`waitForConnected()`函数等待连接的建立。如果连接成功,则表示该端口开放;否则,表示该端口关闭。
运行代码后,程序将会在控制台输出每个扫描到的开放端口信息。请注意,由于多线程同时执行扫描操作,可能会对局域网造成一定的网络负担,请谨慎使用。
阅读全文
相关推荐







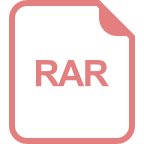



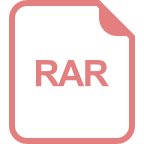







