QT用多线程实现IP端口扫描程序,并输出在线主机
时间: 2024-02-06 09:09:39 浏览: 23
好的,我可以给你提供一个基本的思路。
首先,你需要定义一个IP地址范围,以及要扫描的端口范围。接下来,你需要创建多个线程,每个线程负责扫描一段IP地址。
在每个线程中,你需要使用socket库来连接每个IP地址和端口号,如果连接成功,则表示该主机在线,并将其输出。
以下是一个简单的示例代码:
```cpp
#include <QCoreApplication>
#include <QThread>
#include <QTcpSocket>
#include <QDebug>
const int THREAD_NUM = 10; // 创建10个线程
const int PORT_START = 1; // 扫描端口从1开始
const int PORT_END = 65535; // 扫描端口到65535结束
class ScanThread : public QThread
{
public:
ScanThread(const QString &ip, int portStart, int portEnd)
: m_ip(ip), m_portStart(portStart), m_portEnd(portEnd)
{
}
protected:
void run() override
{
for (int port = m_portStart; port <= m_portEnd; ++port) {
QTcpSocket sock;
sock.connectToHost(m_ip, port);
if (sock.waitForConnected(100)) {
qDebug() << m_ip << ":" << port << "is open.";
}
sock.close();
}
}
private:
QString m_ip;
int m_portStart;
int m_portEnd;
};
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
QStringList ips;
ips << "192.168.1.1/24" << "192.168.2.1/24" << "192.168.3.1/24"; // 定义要扫描的IP地址范围
QList<ScanThread*> threads;
for (const auto& ip : ips) {
for (int i = 0; i < THREAD_NUM; ++i) {
threads.append(new ScanThread(ip, PORT_START + i * (PORT_END - PORT_START) / THREAD_NUM,
PORT_START + (i + 1) * (PORT_END - PORT_START) / THREAD_NUM));
}
}
for (auto thread : threads) {
thread->start();
}
for (auto thread : threads) {
thread->wait();
}
return a.exec();
}
```
在这个示例代码中,我们定义了要扫描的IP地址范围,然后创建了多个线程来扫描每个IP地址和端口号。每个线程都会连接指定IP地址和端口号,如果连接成功,则将其输出。注意,在每个线程中我们使用了`waitForConnected`函数来等待连接成功,如果超时则表示该端口不可达。
希望这个示例代码可以帮助你完成IP端口扫描程序。
相关推荐
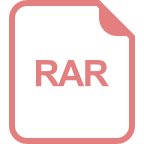
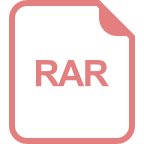














