LocalDateTime处理增加小时数
时间: 2023-11-14 10:08:47 浏览: 55
可以使用 LocalDateTime 的 plusHours 方法来增加小时数。示例代码如下:
```
LocalDateTime now = LocalDateTime.now();
LocalDateTime later = now.plusHours(3);
```
这样,later 就是比 now 晚 3 小时的时间。
相关问题
LocalDateTime
LocalDateTime 是 Java 8 中的一个类,用于表示带时区信息的日期和时间。
可以使用 LocalDateTime 类来表示一个特定的和时间,例如:年、月、日、时、分、秒。它提供了各方法来操作和处理日期时间。
以下是一些常用的 LocalDateTime 操作示例:
1. 创建 LocalDateTime 对象:
```java
LocalDateTime now = LocalDateTime.now(); // 当前日期和时间
LocalDateTime specificDateTime = LocalDateTime.of(2022, 10, 31, 12, 30, 0); // 指定日期和时间
```
2. 获取日期和时间的各个部分:
```java
int year = now.getYear();
Month month = now.getMonth();
int dayOfMonth = now.getDayOfMonth();
int hour = now.getHour();
int minute = now.getMinute();
int second = now.getSecond();
```
3. 修改日期和时间的各个部分:
```java
LocalDateTime modifiedDateTime = now.withYear(2023).withMonth(5).withDayOfMonth(15); // 修改年、月、日
LocalDateTime modifiedDateTime = now.withHour(8).withMinute(45).withSecond(30); // 修改时、分、秒
```
4. 增加或减少日期和时间:
```java
LocalDateTime plusOneDay = now.plusDays(1); // 增加一天
LocalDateTime minusOneHour = now.minusHours(1); // 减少一小时
```
5. 格式化日期和时间输出:
```java
String formattedDateTime = now.format(DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss"));
System.out.println(formattedDateTime);
```
这些只是 LocalDateTime 类的一些基本操作示例,你可以根据自己的需求和业务逻辑进行更多的操作和处理。
LocalDateTime线程安全问题
LocalDateTime是Java 8中引入的日期时间类,它提供了处理日期和时间的方法。在多线程环境下,LocalDateTime是线程安全的,因为它是不可变的(immutable)。
不可变对象是指一旦创建就不能被修改的对象。在多线程环境下,如果多个线程同时访问一个不可变对象,不会出现竞态条件(race condition)或数据不一致的问题。因为不可变对象的状态是固定的,不会被修改。
在LocalDateTime中,所有的方法都是返回一个新的实例,而不是修改原有实例的值。例如,通过plusHours()方法增加小时数,会返回一个新的LocalDateTime实例,而不会修改原有实例的值。这样就保证了线程安全性。
总结一下,LocalDateTime是线程安全的,因为它是不可变的。在多线程环境下,可以放心地使用LocalDateTime对象而不用担心线程安全问题。
相关推荐
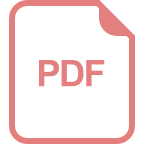
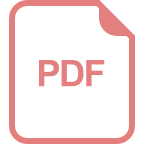







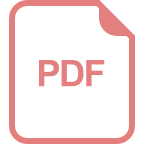
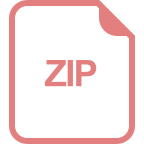