js实现继承的两种方式
时间: 2023-11-28 16:05:09 浏览: 42
JavaScript 实现继承的两种常用方式是原型链继承和构造函数继承。
1. 原型链继承:
原型链继承是 JavaScript 中最基本的继承方式。通过将父类的实例赋值给子类的原型,使子类能够继承父类的属性和方法。具体实现步骤如下:
```javascript
function Parent() {
this.name = 'Parent';
}
Parent.prototype.sayHello = function() {
console.log('Hello, I am ' + this.name);
};
function Child() {
this.name = 'Child';
}
Child.prototype = new Parent(); // 将 Parent 的实例赋值给 Child 的原型
var child = new Child();
child.sayHello(); // 输出: Hello, I am Child
```
2. 构造函数继承:
构造函数继承是通过在子类的构造函数中调用父类的构造函数来实现继承。在子类的构造函数中使用 `call` 或 `apply` 方法来调用父类的构造函数,从而实现属性的继承。具体实现步骤如下:
```javascript
function Parent() {
this.name = 'Parent';
}
Parent.prototype.sayHello = function() {
console.log('Hello, I am ' + this.name);
};
function Child() {
Parent.call(this); // 调用父类的构造函数
this.name = 'Child';
}
var child = new Child();
child.sayHello(); // 输出: Hello, I am Child
```
以上两种方式各有优缺点,原型链继承会导致多个实例共享同一个父类的实例属性,而构造函数继承只能继承父类的实例属性,无法继承父类的原型属性。在实际应用中,可以根据需求选择适合的继承方式。
相关推荐
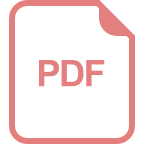
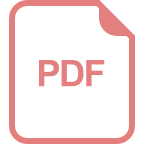
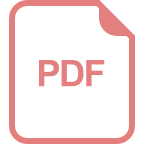














