用VC++ 6.0 设计一个线性表,采用顺序存储结构,插入一个元素的代码
时间: 2024-10-10 07:08:53 浏览: 52
在 VC++ 6.0 中设计一个简单的顺序存储线性表,比如动态数组,插入一个元素的步骤可以如下:
首先,我们需要包含必要的头文件,并定义一个节点的数据结构和线性表类。
```cpp
// Node.h
#pragma once
struct Node {
int data;
Node* next;
};
// List.h
class LinearList {
private:
Node* head; // 首节点指针
int size; // 列表长度
public:
LinearList(); // 构造函数
~LinearList(); // 析构函数
void insert(int value); // 插入元素的方法
};
```
接下来,在 `List.cpp` 文件中实现插入操作:
```cpp
#include "List.h"
#include <iostream>
// 构造函数和析构函数
LinearList::LinearList() : head(nullptr), size(0) {}
LinearList::~LinearList() {
while (head != nullptr) {
Node* temp = head;
head = head->next;
delete temp;
}
}
// 插入元素
void LinearList::insert(int value) {
Node* newNode = new Node();
newNode->data = value;
newNode->next = nullptr;
if (size == 0) {
head = newNode;
} else {
Node* current = head;
while (current->next != nullptr) {
current = current->next;
}
current->next = newNode;
}
size++;
}
```
这个简单的线性表只支持在列表末尾插入元素。如果需要在特定位置插入,你需要修改 `insert` 函数的逻辑。
阅读全文
相关推荐
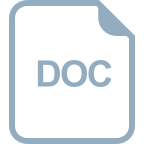
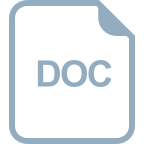
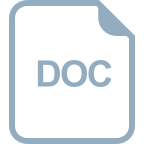
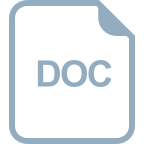

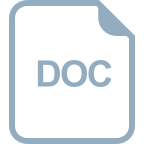
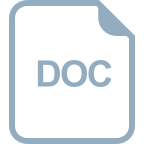
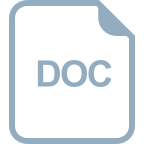
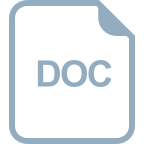
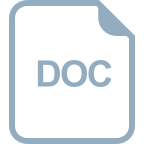
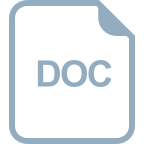
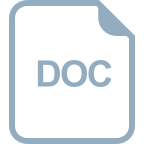
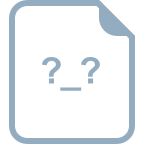
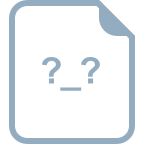
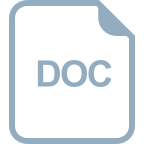
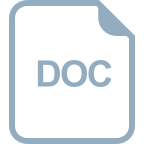
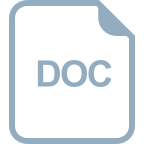
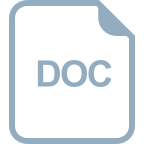
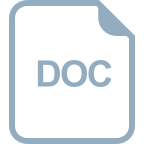