两个整数的四则运算c语言
时间: 2024-10-22 21:15:55 浏览: 37
在C语言中,你可以通过结构化编程来实现两个整数的四则运算,包括加法、减法、乘法和除法。下面是一个简单的示例:
```c
#include <stdio.h>
// 定义结构体来封装两个整数及其操作结果
typedef struct {
int num1; // 第一个整数
int num2; // 第二个整数
int sum; // 加法结果
int difference; // 减法结果
int product; // 乘法结果
int quotient; // 除法结果 (假设num2非零)
} Operation;
// 函数实现四则运算
void perform_arithmetic(Operation* op) {
op->sum = op->num1 + op->num2;
op->difference = op->num1 - op->num2;
op->product = op->num1 * op->num2;
if (op->num2 != 0) { // 避免除以零错误
op->quotient = op->num1 / op->num2;
} else {
printf("Error: Division by zero is not allowed.\n");
}
}
int main() {
Operation calc;
int num1, num2;
printf("Enter two integers: ");
scanf("%d %d", &num1, &num2);
// 初始化运算结构体
calc.num1 = num1;
calc.num2 = num2;
// 执行运算并获取结果
perform_arithmetic(&calc);
printf("Sum: %d\n", calc.sum);
printf("Difference: %d\n", calc.difference);
printf("Product: %d\n", calc.product);
if (calc.quotient != INT_MIN) { // 判断是否成功计算了商
printf("Quotient: %d\n", calc.quotient);
}
return 0;
}
```
在这个程序中,我们首先定义了一个结构`Operation`,包含两个输入整数和它们的结果。然后创建了一个函数`perform_arithmetic`,用于计算各个算术结果。在`main`函数中,用户输入两个数字,结构体接收这些值,并在执行完运算后输出结果。
阅读全文
相关推荐







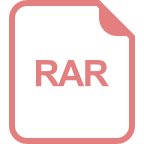










