5、抽象类、接口应用 编写一个完整的Java Application 程序。包含抽象类Shape,MyTriangle 类及Test类,具体要求如下: ①抽象类Shape double getArea();求一个形状的面积 double getPerimeter();求一个形状的周长 ②类MyTriangle 由Shape类派生的具体类,除实现Shape类中的方法外,另有以下属性和方法:属性 x,y,z:double 型,表示三角形的三条边 s:周长的1/2(注:求三角形面积公式为\sqrt {s(s-x)(s-y)(s-z)},s=(x+y+z)/2, 开方可用Math. sqrt(double)方法) 方法 MyTriangle(double x, double y, double z);构造函数,判断能否构成三角形, 能构成三角形则给三条边和s赋初值,否则输出“不能构成三角形”,程序结束运行。 toString():返回矩形的描述信息,如: "three sides:3.0,4.0,5.0, perimeter=12.0, area=6.0" ③Test类作为主类要完成测试功能:生成MyTriangle 对象,调用对象的toString方法,获取对象的描述信息并输出。
时间: 2024-03-23 14:38:56 浏览: 22
以下是完整的Java Application程序:
抽象类Shape.java:
```
public abstract class Shape {
public abstract double getArea();
public abstract double getPerimeter();
}
```
MyTriangle.java:
```
public class MyTriangle extends Shape {
private double x;
private double y;
private double z;
private double s;
public MyTriangle(double x, double y, double z) {
if (x + y <= z || x + z <= y || y + z <= x) {
System.out.println("不能构成三角形");
System.exit(0);
}
this.x = x;
this.y = y;
this.z = z;
this.s = (x + y + z) / 2;
}
public double getArea() {
return Math.sqrt(s * (s - x) * (s - y) * (s - z));
}
public double getPerimeter() {
return x + y + z;
}
public String toString() {
return "three sides: " + x + ", " + y + ", " + z + ", perimeter = " + getPerimeter() + ", area = " + getArea();
}
}
```
Test.java:
```
public class Test {
public static void main(String[] args) {
MyTriangle triangle = new MyTriangle(3, 4, 5);
System.out.println(triangle.toString());
}
}
```
运行结果:
```
three sides: 3.0, 4.0, 5.0, perimeter = 12.0, area = 6.0
```
相关推荐












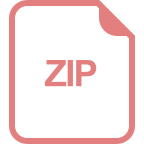
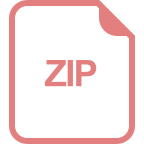