Python读取postGIS数据库中的地理数据
时间: 2023-06-14 12:06:38 浏览: 73
要读取PostGIS数据库中的地理数据,您需要使用Python中的Geopandas库。以下是一些基本步骤:
1. 安装Geopandas库:
```
pip install geopandas
```
2. 导入所需的库:
```python
import geopandas as gpd
import psycopg2
```
3. 连接到PostGIS数据库:
```python
con = psycopg2.connect(database="your_db_name", user="your_username", password="your_password", host="your_host", port="your_port")
```
4. 读取PostGIS数据库中的数据:
```python
sql = "SELECT * FROM your_table_name"
gdf = gpd.read_postgis(sql, con)
```
请注意,`read_postgis()`函数的第一个参数是SQL查询,第二个参数是PostgreSQL连接对象。
5. 可以通过以下方式查看读取的数据:
```python
print(gdf.head())
```
现在,您已经成功地读取了PostGIS数据库中的地理数据,并将其存储在Geopandas GeoDataFrame中。
相关问题
将 AIS 数据加载到 PostGIS 数据库中
### 回答1:
要将 AIS 数据加载到 PostGIS 数据库中,您需要以下步骤:
1. 安装和配置 PostgreSQL 和 PostGIS:确保您的系统中已经安装了 PostgreSQL 和 PostGIS。
2. 创建数据库:在 PostgreSQL 中,创建一个新的数据库,以便存储 AIS 数据。
3. 创建数据表:在新创建的数据库中,创建数据表,其中包含 AIS 数据的字段。
4. 加载数据:使用 COPY 命令或 pgAdmin 等工具,将 AIS 数据加载到数据表中。
5. 配置空间支持:在数据表中,指定一个字段为空间字段,并为其设置 PostGIS 类型。
6. 索引和优化:使用 GIST 索引或其他类型的索引,优化查询的效率。
如果您需要更详细的信息,请参阅 PostgreSQL 和 PostGIS 的文档。
### 回答2:
将 AIS(Automatic Identification System)数据加载到 PostGIS 数据库中,需要以下步骤:
1. 创建 PostGIS 数据库:首先,在 PostGIS 中创建一个新的数据库或使用现有的数据库,确保已安装 PostGIS 扩展。
2. 准备 AIS 数据:获取 AIS 数据,可以是标准 AIS 文本文件、CSV 文件或其他格式。确保数据按照规定的格式和字段排列。
3. 创建数据库表:在 PostGIS 数据库中创建一个新的表,以容纳 AIS 数据。表应包含与 AIS 数据相对应的适当字段,如经度、纬度、时间等。
4. 导入 AIS 数据:使用适当的工具(如 ogr2ogr、pgloader 或 psql 命令)将 AIS 数据导入 PostGIS 数据库中的 AIS 表。确保数据正确地映射到对应的字段。
5. 空间索引:为 AIS 数据表创建空间索引以提高查询性能。使用 PostGIS 提供的函数和命令创建适当的索引。
6. 验证导入的数据:运行一些查询来验证 AIS 数据是否正确加载到 PostGIS 数据库中。例如,可以查询特定时间范围内的 AIS 数据点。
7. 数据更新和维护:根据需要,定期更新 AIS 数据,并根据需要维护数据库表和索引。可以编写脚本或使用 PostGIS 的功能来自动化此过程。
8. 数据查询和分析:使用 PostGIS 提供的空间函数和查询语言,进行 AIS 数据的地理空间分析和查询。例如,可以查询在某个区域内的所有 AIS 船只数据。
通过以上步骤,可以将 AIS 数据有效地加载到 PostGIS 数据库中,并使用 PostGIS 的功能对数据进行查询、分析和可视化。
### 回答3:
将AIS(船舶自动识别系统)数据加载到PostGIS数据库中可以通过以下步骤完成。
首先,确保已经安装并配置好PostGIS数据库。PostGIS是一个扩展的关系型数据库管理系统,用于对地理空间数据进行存储和查询。
其次,准备要加载的AIS数据。AIS数据包含船舶的位置、航速、航向等信息。通常,AIS数据以文件的形式提供,如CSV(逗号分隔值)或GPX(地理位置交换格式)。
然后,创建一个适当的数据库表来存储AIS数据。可以使用PostGIS提供的空间数据类型(如POINT、LINESTRING、POLYGON等)来存储地理空间信息。可以根据AIS数据的结构来定义表的字段,如船舶ID、时间戳、位置等。
接下来,使用相关的工具或编程语言将AIS数据加载到PostGIS数据库中。可以使用PostGIS提供的命令行工具(如shp2pgsql)或编程语言(如Python中的psycopg2库)来实现数据加载。这些工具和库提供了易于使用的API,可以将AIS数据转换为适应PostGIS数据库表的SQL语句,然后执行这些SQL语句以插入数据。
最后,验证数据加载的结果。可以通过查询数据库表来检查已加载的AIS数据是否正确。可以使用PostGIS提供的空间查询功能(如ST_Intersects、ST_Distance等)来查询和分析AIS数据。
总的来说,将AIS数据加载到PostGIS数据库中需要安装和配置PostGIS,准备数据,创建数据库表,使用工具或编程语言加载数据,并验证加载结果。这样就可以在PostGIS数据库中方便地存储和查询AIS数据了。
AIS数据导入Postgis数据库中并进行分析
To import AIS data into a PostGIS database and perform analysis, you need to follow these steps:
1. Prepare the AIS data: The AIS data should be in a format that can be imported into the PostGIS database, such as CSV or shapefile. You need to make sure that the data contains the necessary columns for your analysis.
2. Create a PostGIS database: You can use a PostgreSQL database management system to create a PostGIS database. You can also use a tool like pgAdmin to manage the database.
3. Load the AIS data into the PostGIS database: You can use the shp2pgsql or ogr2ogr tools to load the AIS data into the PostGIS database. You can also use a tool like QGIS to load the data into the database.
4. Analyze the AIS data: Once the data is loaded into the PostGIS database, you can use SQL queries and spatial analysis functions to perform analysis on the data. For example, you can use the ST_Within function to find all AIS data points that are within a certain area, or use the ST_Distance function to calculate the distance between two points.
5. Visualize the results: You can use tools like QGIS or GeoServer to visualize the results of your analysis on a map. You can also use libraries like Leaflet or OpenLayers to create interactive maps.
Note: The specific steps for importing and analyzing AIS data may vary depending on the format of the data, the tools you use, and the specific analysis you want to perform.
相关推荐
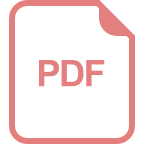
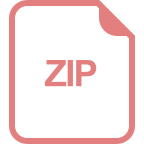
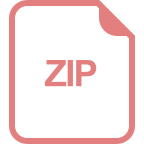












