请用Python语言,利用A*算法完成某路径最短规划问题代码
时间: 2024-10-20 13:19:13 浏览: 25
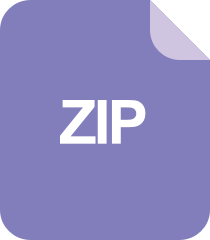
Modeling_and_Simulation2021:[SIAE] [python] 使用A*算法的最短路径优化设计 (3d)
在Python中,我们可以使用A*算法来解决路径最短规划问题,比如在一个网格地图上寻找从起点到终点的最短路径。以下是基本的A*算法实现,使用`heapq`库来管理优先队列:
```python
import heapq
def heuristic(a, b):
# 这里通常采用曼哈顿距离或欧几里得距离作为启发函数,这里假设是曼哈顿距离
return abs(a[0] - b[0]) + abs(a[1] - b[1])
def a_star_search(graph, start, goal):
frontier = [(0, start)] # 开放列表,初始元素是启发值和起始节点
came_from = {} # 记录每个节点是如何到达的
cost_so_far = {start: 0} # 每条边的成本,从起点开始是0
while frontier:
_, current = heapq.heappop(frontier) # 取出启发值最小的节点
if current == goal:
break
for next in graph.neighbors(current): # 遍历当前节点的所有邻居
new_cost = cost_so_far[current] + graph.cost(current, next)
if next not in cost_so_far or new_cost < cost_so_far[next]:
cost_so_far[next] = new_cost
priority = new_cost + heuristic(goal, next)
heapq.heappush(frontier, (priority, next))
path = []
if goal in came_from:
path.append(goal)
while path[-1] != start:
path.append(came_from[path[-1]])
return path[::-1] # 返回从目标到起点的路径
# 例子:假设我们有一个简单的二维网格地图表示
graph = {
'A': {'B': 1},
'B': {'C': 1, 'D': 1},
'C': {},
'D': {'E': 1}
}
# 使用A*搜索从'A'到'E'
print(a_star_search(graph, 'A', 'E')) # 输出路径,如['A', 'B', 'D', 'E']
```
在这个例子中,你需要为地图定义`neighbors()`和`cost()`方法,分别返回给定节点的相邻节点和两个节点之间的成本。
阅读全文
相关推荐
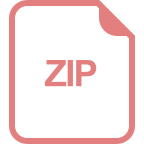
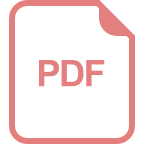
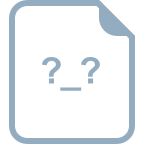
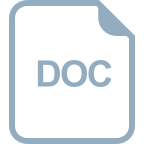
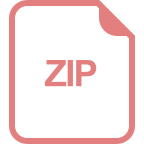
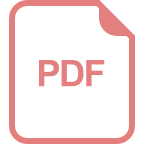
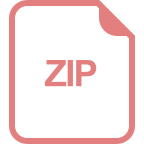
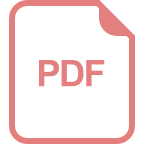
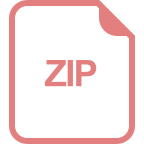
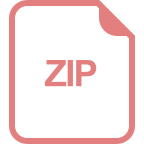
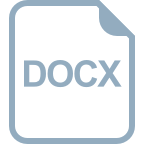
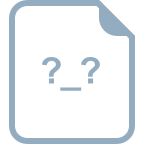
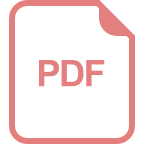
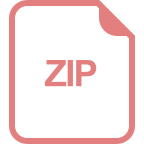

