c++实现空间一点p的坐标为(x,y,z),其中x,y,z为整数。编写点类Point3D,定义空间两点之间的加”+”,减”-”运算为相应三个坐标值分别进行加、减运算,要求实现空间两点之间的加”+”减”-”赋值”=”运算,空间两点间的比较”= =”运算。要求编写Point3D类的声明定义和测试程序
时间: 2024-02-21 09:59:06 浏览: 12
以下是Point3D类的声明和定义:
```cpp
class Point3D {
public:
Point3D(int x = 0, int y = 0, int z = 0); // 构造函数
Point3D operator+(const Point3D& p) const; // 加运算
Point3D operator-(const Point3D& p) const; // 减运算
Point3D& operator=(const Point3D& p); // 赋值运算
bool operator==(const Point3D& p) const; // 相等运算
int x() const; // 获取x坐标
int y() const; // 获取y坐标
int z() const; // 获取z坐标
private:
int m_x, m_y, m_z;
};
Point3D::Point3D(int x, int y, int z)
: m_x(x), m_y(y), m_z(z)
{
}
Point3D Point3D::operator+(const Point3D& p) const
{
return Point3D(m_x + p.m_x, m_y + p.m_y, m_z + p.m_z);
}
Point3D Point3D::operator-(const Point3D& p) const
{
return Point3D(m_x - p.m_x, m_y - p.m_y, m_z - p.m_z);
}
Point3D& Point3D::operator=(const Point3D& p)
{
m_x = p.m_x;
m_y = p.m_y;
m_z = p.m_z;
return *this;
}
bool Point3D::operator==(const Point3D& p) const
{
return m_x == p.m_x && m_y == p.m_y && m_z == p.m_z;
}
int Point3D::x() const
{
return m_x;
}
int Point3D::y() const
{
return m_y;
}
int Point3D::z() const
{
return m_z;
}
```
以下是测试程序:
```cpp
#include <iostream>
using namespace std;
int main() {
Point3D p1(1, 2, 3);
Point3D p2(4, 5, 6);
// 加
Point3D p3 = p1 + p2;
cout << "(" << p3.x() << ", " << p3.y() << ", " << p3.z() << ")" << endl;
// 减
Point3D p4 = p1 - p2;
cout << "(" << p4.x() << ", " << p4.y() << ", " << p4.z() << ")" << endl;
// 赋值
p1 = p2;
cout << "(" << p1.x() << ", " << p1.y() << ", " << p1.z() << ")" << endl;
// 相等
if (p1 == p2) {
cout << "p1 == p2" << endl;
} else {
cout << "p1 != p2" << endl;
}
return 0;
}
```
输出结果为:
```
(5, 7, 9)
(-3, -3, -3)
(4, 5, 6)
p1 != p2
```
相关推荐
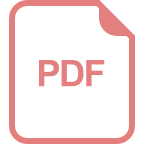
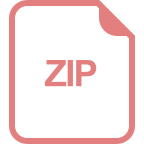
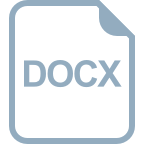











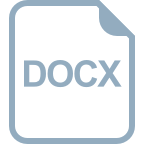
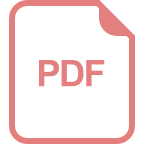
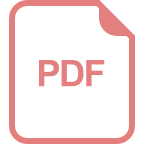
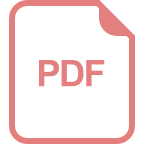