自定义一个注解,用它标记的执行并于给定值做对比(即简单的junit)
时间: 2024-03-02 09:54:28 浏览: 15
好的,可以使用Java中的注解来实现类似于Junit的测试框架。首先自定义一个注解,可以定义在方法或类上,用于标记需要测试的方法或类:
```java
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
@Retention(RetentionPolicy.RUNTIME)
@Target({ElementType.METHOD, ElementType.TYPE})
public @interface MyTest {
String value() default "";
}
```
在注解上使用@Retention和@Target注解来指定注解的生命周期和作用目标。这里设定注解的生命周期为RUNTIME,表示可以在程序运行时读取该注解信息。作用目标包括方法和类。
接着,编写一个测试框架类,用于执行被@MyTest注解标记的方法:
```java
import java.lang.reflect.Method;
public class MyTestFramework {
public static void runTests(Class<?> clazz) {
Method[] methods = clazz.getDeclaredMethods();
for (Method method : methods) {
if (method.isAnnotationPresent(MyTest.class)) {
try {
method.invoke(null);
System.out.println(method.getName() + " passed.");
} catch (Exception e) {
System.out.println(method.getName() + " failed: " + e.getCause());
}
}
}
}
}
```
该测试框架使用Java的反射机制,遍历被测试类中的所有方法,如果某个方法被@MyTest注解标记,则执行该方法并输出测试结果。
最后,编写一个测试类,使用@MyTest注解标记需要测试的方法:
```java
public class MyTestClass {
@MyTest("testAddition")
public static void testAddition() {
int result = 1 + 2;
if (result != 3) {
throw new RuntimeException("Wrong result: " + result);
}
}
@MyTest("testSubtraction")
public static void testSubtraction() {
int result = 5 - 3;
if (result != 2) {
throw new RuntimeException("Wrong result: " + result);
}
}
}
```
在该测试类中,使用@MyTest注解标记了两个方法,分别测试加法和减法的结果是否正确。
最后,可以在main方法中调用测试框架执行测试:
```java
public class Main {
public static void main(String[] args) {
MyTestFramework.runTests(MyTestClass.class);
}
}
```
输出结果为:
```
testAddition passed.
testSubtraction passed.
```
这样就实现了一个简单的测试框架,可以在被@MyTest注解标记的方法上进行测试,并输出测试结果。
相关推荐
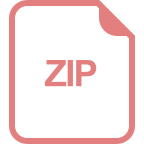
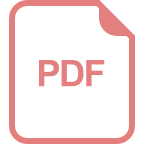
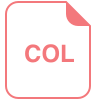
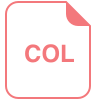
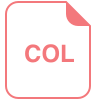
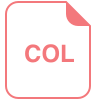
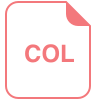









