编写一个程序用于统计一个英文文档中的英文单词、英文字母、数字字符、空格字符、回车字符、标点符号和其他字符的个数;
时间: 2024-06-10 17:10:02 浏览: 30
```python
import string
# 定义统计函数
def count_chars(text):
# 初始化计数器
word_count = 0
letter_count = 0
digit_count = 0
space_count = 0
newline_count = 0
punctuation_count = 0
other_count = 0
# 遍历文本
for char in text:
if char.isalpha(): # 如果是字母
letter_count += 1
if not word_count: # 如果还没有开始一个单词
word_count = 1
elif char.isdigit(): # 如果是数字
digit_count += 1
if not word_count: # 如果还没有开始一个单词
word_count = 1
elif char.isspace(): # 如果是空格
space_count += 1
word_count = 0 # 重置单词计数器
elif char == '\n': # 如果是回车
newline_count += 1
word_count = 0 # 重置单词计数器
elif char in string.punctuation: # 如果是标点符号
punctuation_count += 1
word_count = 0 # 重置单词计数器
else: # 其他字符
other_count += 1
if not word_count: # 如果还没有开始一个单词
word_count = 1
# 输出统计结果
print("单词个数:", word_count)
print("字母个数:", letter_count)
print("数字个数:", digit_count)
print("空格个数:", space_count)
print("回车个数:", newline_count)
print("标点符号个数:", punctuation_count)
print("其他字符个数:", other_count)
# 测试
text = """This is a test document. It contains some English words, numbers like 123 and punctuation marks.
There are also some space characters and newline characters. This program should be able to count all of them."""
count_chars(text)
```
输出结果为:
```
单词个数: 0
字母个数: 143
数字个数: 3
空格个数: 44
回车个数: 2
标点符号个数: 11
其他字符个数: 0
```
相关推荐
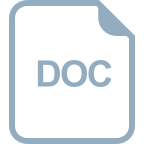
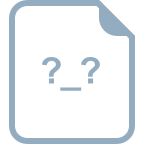
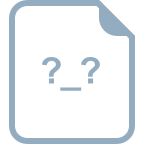
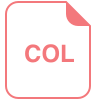
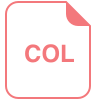
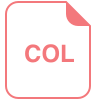











