统计英文字母,空格或换行、数字字符:输入一个正整数n,再输入n个字符,统计其中英文字母、空格或回车、数字字符和其他字符个数
时间: 2024-10-28 17:06:53 浏览: 30
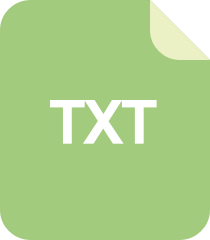
设有n个正整数,将他们连接成一排,组成一个最大的多位整数
要统计输入字符串中的英文字母、空格/回车、数字字符以及其他字符的数量,你可以使用C语言编写一个简单的程序。这里有一个基本的实现思路:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
int n, count_alpha = 0, count_space = 0, count_digit = 0, count_other = 0;
// 获取输入的正整数n
printf("Enter a positive integer n: ");
scanf("%d", &n);
getchar(); // 消耗掉回车符
char input[100];
fgets(input, sizeof(input), stdin); // 读取一行输入
for (size_t i = 0; i < strlen(input); i++) {
if (isalpha(input[i])) { // 判断是否为字母
count_alpha++;
} else if (ispunct(input[i]) || isspace(input[i])) { // 包括空格、换行符和标点符号
if (isspace(input[i])) {
count_space++;
}
// 因为ispunct和isspace都包含了换行符,所以这里不需要额外判断
} else if (isdigit(input[i])) { // 判断是否为数字
count_digit++;
} else { // 其他字符(如特殊符号)
count_other++;
}
}
printf("Number of letters: %d\n", count_alpha);
printf("Number of spaces and newlines: %d\n", count_space);
printf("Number of digits: %d\n", count_digit);
printf("Number of other characters: %d\n", count_other);
return 0;
}
```
在这个程序中,我们使用`ctype.h`库中的`isalpha()`、`ispunct()`、`isspace()`和`isdigit()`函数来检查每个字符。`isalpha()`用于判断是否为字母,`ispunct()`和`isspace()`组合起来检测空格和换行符,`isdigit()`用于识别数字。其余不是这三种类型的字符都被视为其他字符。
阅读全文
相关推荐
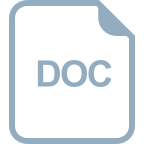
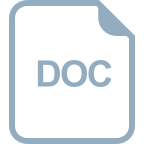















