linux spi读取flashid
时间: 2023-10-17 20:13:24 浏览: 47
在Linux系统中,可以使用spidev驱动程序来进行SPI通信。下面是一个示例代码,用于读取SPI FLASH的ID:
```c
#include <stdio.h>
#include <stdlib.h>
#include <fcntl.h>
#include <unistd.h>
#include <sys/ioctl.h>
#include <linux/spi/spidev.h>
#define SPI_DEVICE "/dev/spidev0.0"
int main(int argc, char *argv[])
{
int fd;
int ret;
uint8_t tx[4], rx[4];
struct spi_ioc_transfer tr = {
.tx_buf = (unsigned long)tx,
.rx_buf = (unsigned long)rx,
.len = 4,
.delay_usecs = 0,
.speed_hz = 1000000,
.bits_per_word = 8,
};
fd = open(SPI_DEVICE, O_RDWR);
if (fd < 0) {
perror("can't open device");
return 1;
}
// 发送读取Flash ID的命令
tx[0] = 0x9F;
tx[1] = 0x00;
tx[2] = 0x00;
tx[3] = 0x00;
ret = ioctl(fd, SPI_IOC_MESSAGE(1), &tr);
if (ret < 1) {
perror("can't send spi message");
return 1;
}
// 输出Flash ID
printf("Flash ID: %02x %02x %02x\n", rx[1], rx[2], rx[3]);
close(fd);
return 0;
}
```
该代码首先打开SPI设备文件,然后发送读取Flash ID的命令,最后输出Flash ID。需要注意的是,Flash ID在rx[1]、rx[2]和rx[3]中,而rx[0]是读取状态寄存器的值。
相关推荐
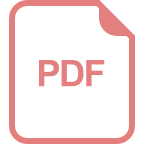
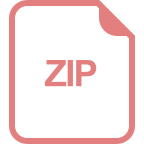














