import numpy as np import pandas as pd import matplotlib.pyplot as plt import pyhht # 读取csv文件数据 data = pd.read_csv('77.csv', header=None) time = data.iloc[:, 0].values charge = data.iloc[:, 1].values # EMD分解 decomposer = pyhht.EMD(charge) imfs = decomposer.decompose()fig, axs = plt.subplots(nrows=imfs.shape[0]+1, ncols=1, figsize=(10, 15)) axs[0].plot(time, charge, label='Original') axs[0].set_xlabel('Time') axs[0].set_ylabel('Charge') axs[0].legend() for i in range(imfs.shape[0]): axs[i+1].plot(time, imfs[i], label=f'IMF{i+1}') axs[i+1].set_xlabel('Time') axs[i+1].set_ylabel('Charge') axs[i+1].legend() residuals = charge - np.sum(imfs, axis=0) axs[imfs.shape[0]+1].plot(time, residuals, label='Residuals') axs[imfs.shape[0]+1].set_xlabel('Time') axs[imfs.shape[0]+1].set_ylabel('Charge') axs[imfs.shape[0]+1].legend() axs[0].set_title('Original Signal') for i in range(imfs.shape[0]): axs[i+1].set_title(f'IMF {i+1}') axs[imfs.shape[0]+1].set_title('Residuals') plt.tight_layout() plt.show()以上代码运行时报出以下错误IndexError: index 4 is out of bounds for axis 0 with size 请修改
时间: 2023-11-28 09:51:39 浏览: 145
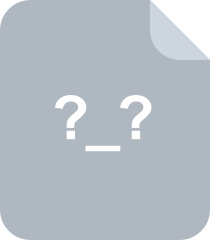
import numpy as np import matplotlib.pyplot as plt import math
可以尝试检查一下数据的维度,看看是否符合预期。同时也可以将代码中的行数和列数调整一下,确保能够正确显示图像。示例代码如下:
```python
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
import pyhht
# 读取csv文件数据
data = pd.read_csv('77.csv', header=None)
time = data.iloc[:, 0].values
charge = data.iloc[:, 1].values
# EMD分解
decomposer = pyhht.EMD()
imfs = decomposer.decompose(charge)
fig, axs = plt.subplots(nrows=imfs.shape[0]+2, ncols=1, figsize=(10, 15))
axs[0].plot(time, charge, label='Original')
axs[0].set_xlabel('Time')
axs[0].set_ylabel('Charge')
axs[0].legend()
for i in range(imfs.shape[0]):
axs[i+1].plot(time, imfs[i], label=f'IMF{i+1}')
axs[i+1].set_xlabel('Time')
axs[i+1].set_ylabel('Charge')
axs[i+1].legend()
residuals = charge - np.sum(imfs, axis=0)
axs[imfs.shape[0]+1].plot(time, residuals, label='Residuals')
axs[imfs.shape[0]+1].set_xlabel('Time')
axs[imfs.shape[0]+1].set_ylabel('Charge')
axs[imfs.shape[0]+1].legend()
axs[0].set_title('Original Signal')
for i in range(imfs.shape[0]):
axs[i+1].set_title(f'IMF {i+1}')
axs[imfs.shape[0]+1].set_title('Residuals')
plt.tight_layout()
plt.show()
```
另外需要注意的是,EMD分解需要先初始化一个EMD对象,然后再调用decompose方法进行处理,因此示例代码中对EMD对象的初始化进行了修正。
阅读全文
相关推荐
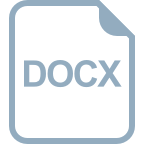
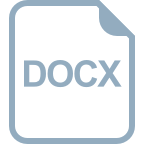















