C语言实现:读取一个文件a.txt,文件中每一行都是一个int数据,逐行读取这些数据,存放在数组中,要求数组中不能够出现重复的数据。
时间: 2024-05-16 12:15:38 浏览: 50
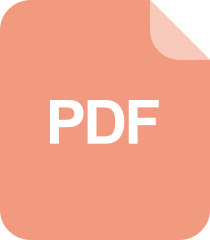
c语言实现把文件中数据读取并存到数组中

可以使用哈希表来实现去重功能,具体步骤如下:
1. 定义一个哈希表,用于存储已经读取的数据。
2. 打开文件a.txt,逐行读取数据,将每个数据插入到哈希表中。
3. 如果插入操作返回false,则说明已经存在相同的数据,直接跳过。
4. 关闭文件a.txt,将哈希表中的数据复制到一个数组中。
下面是具体的C语言实现代码:
```c
#include <stdio.h>
#include <stdbool.h>
#include <stdlib.h>
#include <string.h>
#define TABLE_SIZE 1000
typedef struct HashNode {
int key;
struct HashNode *next;
} HashNode;
typedef struct HashTable {
HashNode *table[TABLE_SIZE];
} HashTable;
HashTable *create_hash_table() {
HashTable *hash_table = (HashTable *)malloc(sizeof(HashTable));
memset(hash_table, 0, sizeof(HashTable));
return hash_table;
}
bool hash_table_insert(HashTable *hash_table, int key) {
int index = abs(key) % TABLE_SIZE;
HashNode *node = hash_table->table[index];
while (node != NULL) {
if (node->key == key) {
return false;
}
node = node->next;
}
node = (HashNode *)malloc(sizeof(HashNode));
node->key = key;
node->next = hash_table->table[index];
hash_table->table[index] = node;
return true;
}
void copy_hash_table_to_array(HashTable *hash_table, int *array, int *size) {
*size = 0;
for (int i = 0; i < TABLE_SIZE; i++) {
HashNode *node = hash_table->table[i];
while (node != NULL) {
array[*size] = node->key;
(*size)++;
node = node->next;
}
}
}
int main() {
FILE *fp = fopen("a.txt", "r");
if (fp == NULL) {
printf("Failed to open file.\n");
return 0;
}
HashTable *hash_table = create_hash_table();
int key;
while (fscanf(fp, "%d", &key) != EOF) {
if (hash_table_insert(hash_table, key)) {
printf("Insert %d successfully.\n", key);
} else {
printf("Duplicate key %d.\n", key);
}
}
fclose(fp);
int size;
int *array = (int *)malloc(sizeof(int) * TABLE_SIZE);
copy_hash_table_to_array(hash_table, array, &size);
for (int i = 0; i < size; i++) {
printf("%d ", array[i]);
}
printf("\n");
free(array);
return 0;
}
```
阅读全文
相关推荐
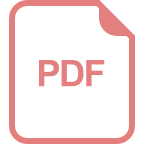
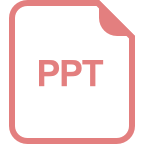
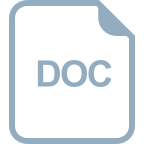
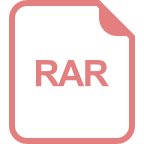
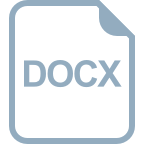
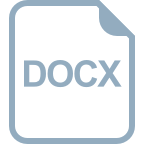
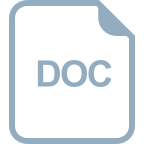
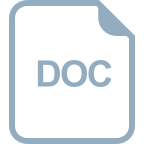
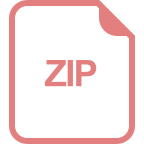
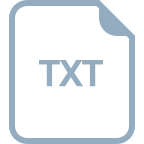
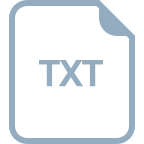
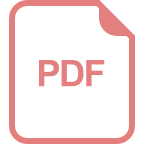
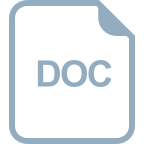
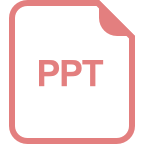
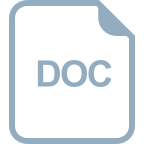