python 依存句法分析
时间: 2023-06-22 21:37:56 浏览: 180
Python的依存句法分析可以使用多个自然语言处理工具进行实现,以下是其中的几个常用工具和方法:
1. Stanford CoreNLP
Stanford CoreNLP是一个流行的Java自然语言处理工具包,可以用于依存句法分析、命名实体识别、句子分割等任务。Python可以通过pycorenlp包与Stanford CoreNLP进行交互,使用方法如下:
```python
from pycorenlp import StanfordCoreNLP
nlp = StanfordCoreNLP('http://localhost:9000')
text = 'John is eating a pizza'
output = nlp.annotate(text, properties={
'annotators': 'depparse',
'outputFormat': 'json'
})
print(output['sentences'][0]['enhancedPlusPlusDependencies'])
```
2. spaCy
spaCy是一个流行的Python自然语言处理库,其依存句法分析器可以通过调用nlp对象的`parse`方法进行实现,使用方法如下:
```python
import spacy
nlp = spacy.load('en_core_web_sm')
text = 'John is eating a pizza'
doc = nlp(text)
for token in doc:
print(token.text, token.dep_, token.head.text, token.head.pos_,
[child for child in token.children])
```
3. nltk
nltk是Python中自然语言处理的老牌库,其也提供了依存句法分析的功能,可以通过`nltk.parse.dependencygraph.DependencyGraph`类进行实现,使用方法如下:
```python
import nltk
text = 'John is eating a pizza'
tokens = nltk.word_tokenize(text)
pos_tags = nltk.pos_tag(tokens)
dependency_parser = nltk.parse.corenlp.CoreNLPDependencyParser()
parse_result = dependency_parser.raw_parse(text)
dependency_graph = parse_result.__next__()
for node in dependency_graph.nodes.values():
print(node['word'], node['tag'], node['head'], node['rel'])
```
阅读全文
相关推荐
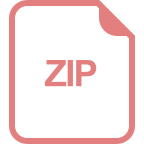

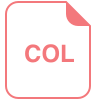
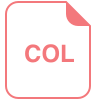


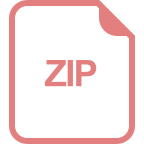
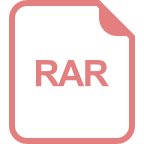
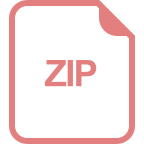
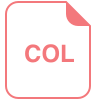
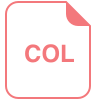
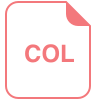
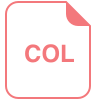
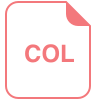

