用C语言写socket ipv6客户端非阻塞方式连接的代码
时间: 2023-10-07 21:07:08 浏览: 144
以下是一个简单的用C语言写的非阻塞式连接IPv6客户端的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <errno.h>
#include <netdb.h>
#include <unistd.h>
#include <fcntl.h>
#include <sys/types.h>
#include <sys/socket.h>
#include <netinet/in.h>
#include <arpa/inet.h>
int main(int argc, char *argv[]) {
int sockfd, flags;
struct addrinfo hints, *res, *p;
int status;
char ipstr[INET6_ADDRSTRLEN];
if (argc != 3) {
fprintf(stderr, "Usage: %s <hostname> <port>\n", argv[0]);
return 1;
}
memset(&hints, 0, sizeof hints);
hints.ai_family = AF_UNSPEC;
hints.ai_socktype = SOCK_STREAM;
if ((status = getaddrinfo(argv[1], argv[2], &hints, &res)) != 0) {
fprintf(stderr, "getaddrinfo error: %s\n", gai_strerror(status));
return 2;
}
for (p = res; p != NULL; p = p->ai_next) {
void *addr;
char *ipver;
if (p->ai_family == AF_INET) { // IPv4
struct sockaddr_in *ipv4 = (struct sockaddr_in *)p->ai_addr;
addr = &(ipv4->sin_addr);
ipver = "IPv4";
} else { // IPv6
struct sockaddr_in6 *ipv6 = (struct sockaddr_in6 *)p->ai_addr;
addr = &(ipv6->sin6_addr);
ipver = "IPv6";
}
// convert the IP to a string and print it:
inet_ntop(p->ai_family, addr, ipstr, sizeof ipstr);
printf("Connecting to %s: %s\n", ipver, ipstr);
sockfd = socket(p->ai_family, p->ai_socktype, p->ai_protocol);
if (sockfd == -1) {
perror("socket");
continue;
}
// set non-blocking mode
flags = fcntl(sockfd, F_GETFL, 0);
if (flags == -1) {
perror("fcntl");
return 3;
}
if (fcntl(sockfd, F_SETFL, flags | O_NONBLOCK) == -1) {
perror("fcntl");
return 3;
}
if (connect(sockfd, p->ai_addr, p->ai_addrlen) == -1) {
if (errno == EINPROGRESS) { // connection in progress
fd_set write_fds;
struct timeval timeout;
FD_ZERO(&write_fds);
FD_SET(sockfd, &write_fds);
timeout.tv_sec = 5; // 5 seconds timeout
timeout.tv_usec = 0;
if (select(sockfd + 1, NULL, &write_fds, NULL, &timeout) > 0) {
int error;
socklen_t len = sizeof error;
if (getsockopt(sockfd, SOL_SOCKET, SO_ERROR, &error, &len) == -1) {
perror("getsockopt");
return 4;
}
if (error != 0) {
fprintf(stderr, "connect error: %s\n", strerror(error));
return 4;
}
} else {
fprintf(stderr, "connect timeout\n");
return 4;
}
} else {
perror("connect");
continue;
}
}
break; // success
}
if (p == NULL) {
fprintf(stderr, "failed to connect\n");
return 5;
}
freeaddrinfo(res);
printf("Connected successfully!\n");
// do something with the socket...
close(sockfd);
return 0;
}
```
该示例使用 `getaddrinfo()` 函数获取主机名和端口号的地址信息,然后尝试连接到每个地址。对于每个地址,我们使用 `socket()` 创建一个套接字,然后将其设置为非阻塞模式。接下来,我们使用 `connect()` 尝试连接到该地址。如果返回 `EINPROGRESS` 错误,则表示连接正在进行中,我们需要使用 `select()` 以非阻塞方式等待连接完成。如果连接超时或出现其他错误,则连接失败。如果连接成功,则我们可以使用该套接字进行通信。最后,我们关闭套接字并返回。
请注意,此代码仅用于示例用途,并不足以处理所有错误和异常情况。
阅读全文
相关推荐

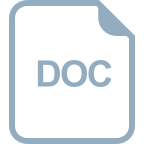
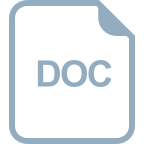
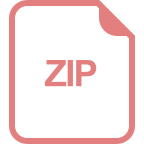
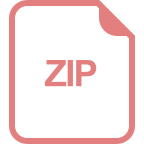
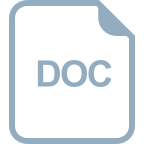
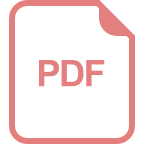
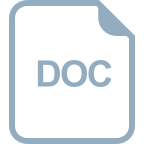
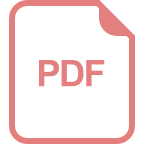
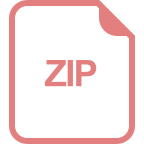
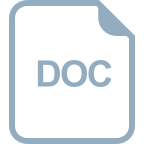
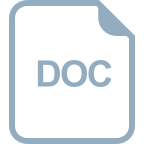
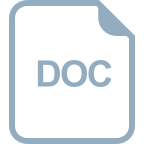
