Java数据结构航空客运订票系统代码
时间: 2024-03-24 10:34:22 浏览: 81
Java数据结构航空客运订票系统代码是一个用Java语言编写的航空客运订票系统的代码实现。该系统使用了多种数据结构来管理航班信息、乘客信息和座位信息等。
以下是一个简单的Java数据结构航空客运订票系统代码示例:
```java
import java.util.HashMap;
import java.util.Map;
public class AirlineTicketSystem {
private Map<String, Flight> flights;
public AirlineTicketSystem() {
flights = new HashMap<>();
}
public void addFlight(String flightNumber, String departure, String destination, int capacity) {
Flight flight = new Flight(flightNumber, departure, destination, capacity);
flights.put(flightNumber, flight);
}
public void bookTicket(String flightNumber, String passengerName) {
Flight flight = flights.get(flightNumber);
if (flight != null) {
if (flight.hasAvailableSeats()) {
flight.bookSeat(passengerName);
System.out.println("Ticket booked successfully.");
} else {
System.out.println("No available seats on this flight.");
}
} else {
System.out.println("Flight not found.");
}
}
public void displayFlightDetails(String flightNumber) {
Flight flight = flights.get(flightNumber);
if (flight != null) {
System.out.println("Flight Number: " + flight.getFlightNumber());
System.out.println("Departure: " + flight.getDeparture());
System.out.println("Destination: " + flight.getDestination());
System.out.println("Available Seats: " + flight.getAvailableSeats());
} else {
System.out.println("Flight not found.");
}
}
private class Flight {
private String flightNumber;
private String departure;
private String destination;
private int capacity;
private int bookedSeats;
public Flight(String flightNumber, String departure, String destination, int capacity) {
this.flightNumber = flightNumber;
this.departure = departure;
this.destination = destination;
this.capacity = capacity;
this.bookedSeats = 0;
}
public String getFlightNumber() {
return flightNumber;
}
public String getDeparture() {
return departure;
}
public String getDestination() {
return destination;
}
public int getAvailableSeats() {
return capacity - bookedSeats;
}
public boolean hasAvailableSeats() {
return bookedSeats < capacity;
}
public void bookSeat(String passengerName) {
bookedSeats++;
System.out.println("Seat booked for passenger: " + passengerName);
}
}
}
```
这段代码实现了一个简单的航空客运订票系统,其中包含了航班信息的添加、乘客订票和显示航班详情等功能。航班信息使用Flight类来表示,每个Flight对象包含航班号、出发地、目的地、座位容量和已预订座位数等属性。航班信息存储在AirlineTicketSystem类中的flights变量中,使用HashMap来进行快速查找。
阅读全文
相关推荐
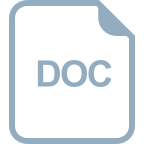
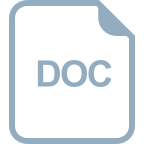
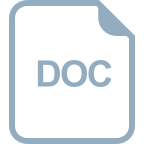
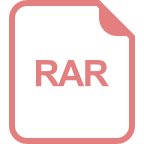
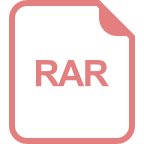
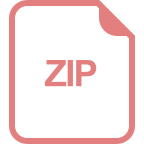
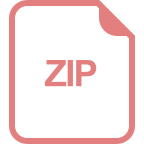
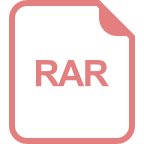
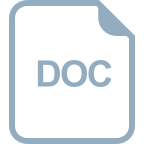

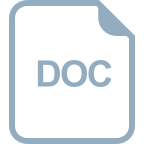
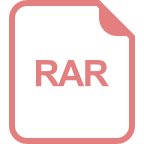
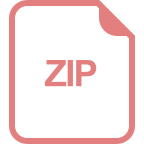
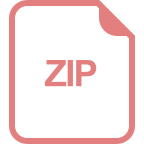
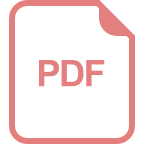
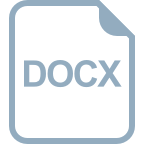
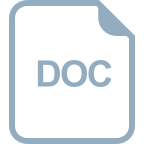
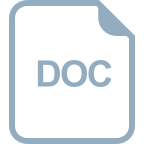