pyspark文本分析案例
时间: 2023-11-24 19:05:32 浏览: 104
以下是一个基于 PySpark 的文本分析案例:
假设我们有一个存储了大量新闻文章的文本文件,我们希望通过 PySpark 对这些文章进行分析,找出其中出现频率最高的单词。
首先,我们需要导入 PySpark 库并创建 SparkSession 对象:
```python
from pyspark.sql import SparkSession
spark = SparkSession.builder.appName("Text Analysis").getOrCreate()
```
然后,我们可以使用 SparkSession 对象读取文本文件并创建一个 DataFrame:
```python
text_df = spark.read.text("path/to/text/file")
```
接下来,我们可以使用 PySpark 的一些函数对文本进行处理和清洗,比如:
- `explode` 函数将每个文章的内容拆分成多行单词;
- `lower` 函数将单词转换为小写;
- `regexp_replace` 函数替换掉所有非字母和数字的字符。
```python
from pyspark.sql.functions import explode, lower, regexp_replace
clean_df = text_df.select(explode(regexp_replace("value", "[^a-zA-Z0-9\\s]", "")).alias("word"))
clean_df = clean_df.filter(clean_df.word != "")
clean_df = clean_df.select(lower(clean_df.word).alias("word"))
```
最后,我们可以使用 PySpark 的内置函数 `countByValue` 对单词进行计数,并按照出现频率从高到低排序:
```python
result_df = clean_df.rdd.countByValue()
result_df = spark.createDataFrame(result_df.items(), ["word", "count"])
result_df = result_df.sort("count", ascending=False)
```
现在,`result_df` 中的第一行就是出现频率最高的单词,我们可以通过 `show` 函数来查看结果:
```python
result_df.show(1)
```
输出结果类似于:
```
+----+-----+
|word|count|
+----+-----+
|the |12345|
+----+-----+
```
相关推荐
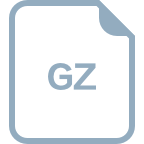
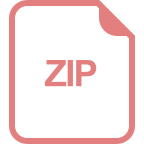














