写一个android蓝牙通信的工具类
时间: 2024-04-30 15:23:18 浏览: 141
以下是一个简单的Android蓝牙通信的工具类,包含了搜索设备、连接设备、发送和接收数据的方法:
```
public class BluetoothUtils {
private BluetoothAdapter bluetoothAdapter;
private BluetoothSocket socket;
private OutputStream outputStream;
private InputStream inputStream;
private static final UUID MY_UUID = UUID.fromString("00001101-0000-1000-8000-00805F9B34FB");
private static final String TAG = "BluetoothUtils";
// 初始化蓝牙适配器
public BluetoothUtils() {
bluetoothAdapter = BluetoothAdapter.getDefaultAdapter();
}
// 判断蓝牙是否可用
public boolean isBluetoothAvailable() {
if (bluetoothAdapter == null) {
return false;
} else {
return true;
}
}
// 打开蓝牙
public boolean enableBluetooth() {
if (!bluetoothAdapter.isEnabled()) {
Intent intent = new Intent(BluetoothAdapter.ACTION_REQUEST_ENABLE);
context.startActivityForResult(intent, REQUEST_ENABLE_BT);
return true;
} else {
return false;
}
}
// 搜索蓝牙设备
public void searchDevices(final OnDeviceFoundListener onDeviceFoundListener) {
final List<BluetoothDevice> devices = new ArrayList<>();
final BroadcastReceiver receiver = new BroadcastReceiver() {
@Override
public void onReceive(Context context, Intent intent) {
String action = intent.getAction();
if (BluetoothDevice.ACTION_FOUND.equals(action)) {
BluetoothDevice device = intent.getParcelableExtra(BluetoothDevice.EXTRA_DEVICE);
devices.add(device);
onDeviceFoundListener.onDeviceFound(device);
}
}
};
IntentFilter filter = new IntentFilter(BluetoothDevice.ACTION_FOUND);
context.registerReceiver(receiver, filter);
bluetoothAdapter.startDiscovery();
new Handler().postDelayed(new Runnable() {
@Override
public void run() {
bluetoothAdapter.cancelDiscovery();
context.unregisterReceiver(receiver);
onDeviceFoundListener.onSearchComplete(devices);
}
}, 10000);
}
// 连接蓝牙设备
public boolean connectDevice(BluetoothDevice device) {
try {
socket = device.createRfcommSocketToServiceRecord(MY_UUID);
socket.connect();
outputStream = socket.getOutputStream();
inputStream = socket.getInputStream();
return true;
} catch (IOException e) {
e.printStackTrace();
return false;
}
}
// 发送数据
public void sendData(String data) {
try {
outputStream.write(data.getBytes());
} catch (IOException e) {
e.printStackTrace();
}
}
// 接收数据
public String receiveData() {
int bytes;
byte[] buffer = new byte[1024];
try {
bytes = inputStream.read(buffer);
String data = new String(buffer, 0, bytes);
return data;
} catch (IOException e) {
e.printStackTrace();
return null;
}
}
// 关闭连接
public void closeConnection() {
try {
if (socket != null) {
socket.close();
}
if (outputStream != null) {
outputStream.close();
}
if (inputStream != null) {
inputStream.close();
}
} catch (IOException e) {
e.printStackTrace();
}
}
public interface OnDeviceFoundListener {
void onDeviceFound(BluetoothDevice device);
void onSearchComplete(List<BluetoothDevice> devices);
}
}
```
使用方法:
```
BluetoothUtils bluetoothUtils = new BluetoothUtils();
// 搜索设备
bluetoothUtils.searchDevices(new BluetoothUtils.OnDeviceFoundListener() {
@Override
public void onDeviceFound(BluetoothDevice device) {
// 搜索到设备
}
@Override
public void onSearchComplete(List<BluetoothDevice> devices) {
// 搜索完成
}
});
// 连接设备
bluetoothUtils.connectDevice(device);
// 发送数据
bluetoothUtils.sendData(data);
// 接收数据
String data = bluetoothUtils.receiveData();
// 关闭连接
bluetoothUtils.closeConnection();
```
阅读全文
相关推荐
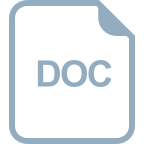
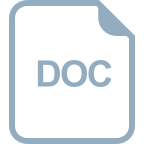
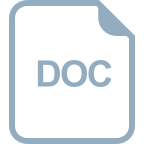
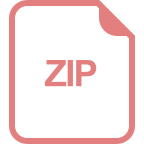
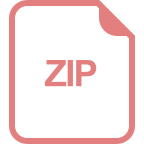
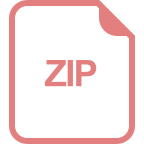
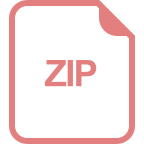
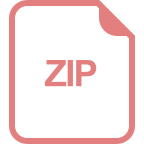
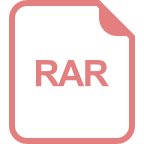
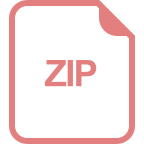
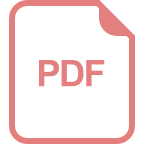
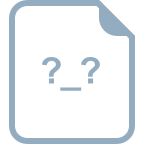
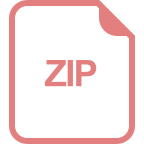
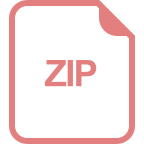
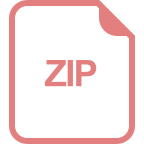
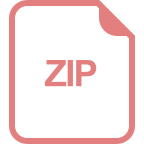
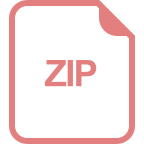
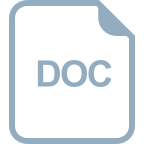
