java实现计时(附完整源码)
时间: 2023-10-17 08:25:46 浏览: 70
以下是Java实现计时的完整源码:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.text.DecimalFormat;
public class TimerDemo extends JFrame implements ActionListener {
private static final long serialVersionUID = 1L;
private Timer timer; // 计时器
private JLabel timeLabel; // 显示时间的标签
private JButton startButton, stopButton, resetButton; // 开始、停止、重置按钮
private long startTime; // 开始时间
private DecimalFormat format; // 时间格式
public TimerDemo() {
setTitle("计时器");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setBounds(100, 100, 300, 200);
getContentPane().setLayout(new BorderLayout());
timeLabel = new JLabel("00:00:00.000", JLabel.CENTER);
timeLabel.setFont(new Font("Dialog", Font.BOLD, 36));
getContentPane().add(timeLabel, BorderLayout.CENTER);
JPanel buttonPanel = new JPanel(new FlowLayout());
startButton = new JButton("开始");
startButton.addActionListener(this);
buttonPanel.add(startButton);
stopButton = new JButton("停止");
stopButton.addActionListener(this);
buttonPanel.add(stopButton);
resetButton = new JButton("重置");
resetButton.addActionListener(this);
buttonPanel.add(resetButton);
getContentPane().add(buttonPanel, BorderLayout.SOUTH);
format = new DecimalFormat("00");
}
@Override
public void actionPerformed(ActionEvent e) {
if (e.getSource().equals(startButton)) { // 开始计时
startTime = System.currentTimeMillis();
timer = new Timer(10, new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
long time = System.currentTimeMillis() - startTime;
int hour = (int) (time / 3600000);
int minute = (int) ((time - hour * 3600000) / 60000);
int second = (int) ((time - hour * 3600000 - minute * 60000) / 1000);
int millisecond = (int) (time - hour * 3600000 - minute * 60000 - second * 1000);
timeLabel.setText(format.format(hour) + ":" + format.format(minute) + ":" + format.format(second) + "." + format.format(millisecond));
}
});
timer.start();
} else if (e.getSource().equals(stopButton)) { // 停止计时
timer.stop();
} else if (e.getSource().equals(resetButton)) { // 重置计时
timeLabel.setText("00:00:00.000");
}
}
public static void main(String[] args) {
TimerDemo timerDemo = new TimerDemo();
timerDemo.setVisible(true);
}
}
```
该程序使用了Java Swing界面库,创建了一个窗口,包含一个显示时间的标签和开始、停止、重置计时的按钮。计时器使用了Java内置的`Timer`类,每10毫秒更新一次时间标签。`startTime`记录了开始计时的时间,计算当前时间时使用了`System.currentTimeMillis()`方法。时间格式使用了`DecimalFormat`类,格式为`00:00:00.000`。
相关推荐













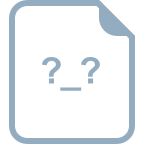