javafx贪吃蛇代码eclipse
时间: 2023-07-09 08:51:39 浏览: 147
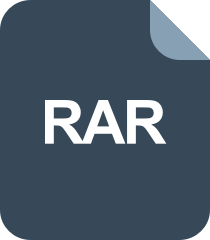
贪吃蛇(java 源码 eclipse项目)

以下是一个使用 JavaFX 编写的贪吃蛇游戏的示例代码:
Snake.java
```java
import javafx.animation.Animation;
import javafx.animation.KeyFrame;
import javafx.animation.Timeline;
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.canvas.Canvas;
import javafx.scene.canvas.GraphicsContext;
import javafx.scene.input.KeyCode;
import javafx.scene.layout.BorderPane;
import javafx.scene.paint.Color;
import javafx.stage.Stage;
import javafx.util.Duration;
public class Snake extends Application {
private static final int WIDTH = 400;
private static final int HEIGHT = 400;
private static final int BLOCK_SIZE = 10;
private int score = 0;
private Direction direction = Direction.RIGHT;
private boolean gameOver = false;
private Timeline timeline;
private SnakeBody head = new SnakeBody(WIDTH / 2, HEIGHT / 2, BLOCK_SIZE, BLOCK_SIZE, Color.GREEN);
private SnakeBody food = createFood();
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) throws Exception {
primaryStage.setTitle("Snake Game");
BorderPane root = new BorderPane();
Canvas canvas = new Canvas(WIDTH, HEIGHT);
GraphicsContext gc = canvas.getGraphicsContext2D();
root.setCenter(canvas);
Scene scene = new Scene(root);
scene.setOnKeyPressed(event -> {
if (event.getCode() == KeyCode.LEFT && direction != Direction.RIGHT) {
direction = Direction.LEFT;
} else if (event.getCode() == KeyCode.RIGHT && direction != Direction.LEFT) {
direction = Direction.RIGHT;
} else if (event.getCode() == KeyCode.UP && direction != Direction.DOWN) {
direction = Direction.UP;
} else if (event.getCode() == KeyCode.DOWN && direction != Direction.UP) {
direction = Direction.DOWN;
}
});
primaryStage.setScene(scene);
primaryStage.show();
timeline = new Timeline(new KeyFrame(Duration.millis(100), event -> {
gc.clearRect(0, 0, WIDTH, HEIGHT);
moveSnake();
drawSnake(gc);
drawFood(gc);
if (gameOver) {
timeline.stop();
showGameOver(gc);
}
}));
timeline.setCycleCount(Animation.INDEFINITE);
timeline.play();
}
private void showGameOver(GraphicsContext gc) {
gc.setFill(Color.RED);
gc.setFont(gc.getFont().getName(), 30);
gc.fillText("Game Over", 100, 150);
gc.fillText("Score: " + score, 120, 200);
}
private void drawFood(GraphicsContext gc) {
gc.setFill(food.getColor());
gc.fillRect(food.getX(), food.getY(), food.getWidth(), food.getHeight());
}
private void drawSnake(GraphicsContext gc) {
SnakeBody body = head;
while (body != null) {
gc.setFill(body.getColor());
gc.fillRect(body.getX(), body.getY(), body.getWidth(), body.getHeight());
body = body.getNext();
}
gc.setFill(Color.BLACK);
gc.fillText("Score: " + score, 10, 20);
}
private void moveSnake() {
int x = head.getX();
int y = head.getY();
switch (direction) {
case LEFT:
x -= BLOCK_SIZE;
break;
case RIGHT:
x += BLOCK_SIZE;
break;
case UP:
y -= BLOCK_SIZE;
break;
case DOWN:
y += BLOCK_SIZE;
break;
}
if (x < 0 || y < 0 || x >= WIDTH || y >= HEIGHT) {
gameOver = true;
return;
}
if (x == food.getX() && y == food.getY()) {
score++;
head = new SnakeBody(x, y, BLOCK_SIZE, BLOCK_SIZE, Color.GREEN, head);
food = createFood();
} else {
SnakeBody newHead = new SnakeBody(x, y, BLOCK_SIZE, BLOCK_SIZE, Color.GREEN, head);
head = newHead;
SnakeBody body = head.getNext();
while (body != null) {
if (head.intersects(body)) {
gameOver = true;
return;
}
body = body.getNext();
}
SnakeBody tail = head;
while (tail.getNext() != null) {
tail = tail.getNext();
}
tail.setNext(null);
}
}
private SnakeBody createFood() {
int x = (int) (Math.random() * (WIDTH / BLOCK_SIZE)) * BLOCK_SIZE;
int y = (int) (Math.random() * (HEIGHT / BLOCK_SIZE)) * BLOCK_SIZE;
return new SnakeBody(x, y, BLOCK_SIZE, BLOCK_SIZE, Color.RED);
}
private enum Direction {
LEFT, RIGHT, UP, DOWN
}
}
```
SnakeBody.java
```java
import javafx.scene.paint.Color;
public class SnakeBody {
private int x;
private int y;
private int width;
private int height;
private Color color;
private SnakeBody next;
public SnakeBody(int x, int y, int width, int height, Color color) {
this.x = x;
this.y = y;
this.width = width;
this.height = height;
this.color = color;
}
public SnakeBody(int x, int y, int width, int height, Color color, SnakeBody next) {
this(x, y, width, height, color);
this.next = next;
}
public int getX() {
return x;
}
public void setX(int x) {
this.x = x;
}
public int getY() {
return y;
}
public void setY(int y) {
this.y = y;
}
public int getWidth() {
return width;
}
public void setWidth(int width) {
this.width = width;
}
public int getHeight() {
return height;
}
public void setHeight(int height) {
this.height = height;
}
public Color getColor() {
return color;
}
public void setColor(Color color) {
this.color = color;
}
public SnakeBody getNext() {
return next;
}
public void setNext(SnakeBody next) {
this.next = next;
}
public boolean intersects(SnakeBody other) {
return x == other.x && y == other.y;
}
}
```
这个游戏的主要逻辑在 `Snake.java` 中,这个类继承自 `Application`,它的 `start()` 方法创建了游戏场景和绑定了键盘事件。游戏的主要逻辑在一个 `Timeline` 中进行,它每隔一段时间检测蛇的移动和游戏是否结束,并根据游戏状态绘制场景。`SnakeBody` 类表示蛇的一个身体部分,包含了位置、大小和颜色等信息。
阅读全文
相关推荐

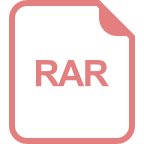
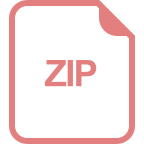
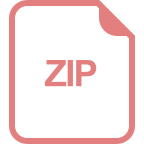
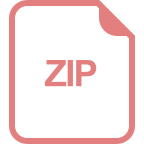
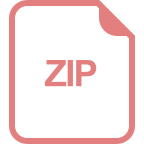
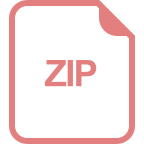
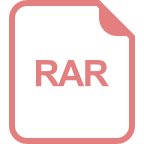
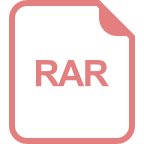
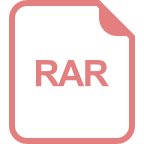
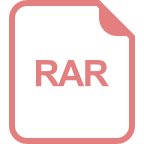
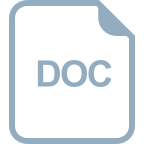
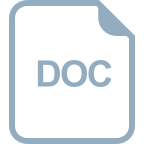

