有一包含100亿条IP访问信息的文本文件,每一行是一个IPv4地址(如:192.168.1.1),要求对文件进行一遍扫描后输出所有出现过的IP地址(不能重复)。按下面三个不同的要求分别给出三个不同的实现类IPProcess1,IPProcess2,IPProcess3。需要实现的接口如下: interface IPProcess{ void process(File in, File out) throws IOException; } 可以添加辅助类,请添加详细的设计说明和注释来解释程序。 IPProcess1: 内存足够大,直接使用HashMap进行数据处理 IPProcess2: 假设可用内存为1G,在内存中实现处理过程。(提示:创建 byte数组,每一位表示一个IP地址,IPV4每位取值范围0-255,可采用位操作) IPProcess3: 假设内存非常少,必须在文件进行操作。(提示:使用RandomAccessFile, 可以使用read(), write(),read(byte[] b) ,write(byte[] b),seek(long pos),getFilePointer()方法 )
时间: 2023-06-16 15:05:13 浏览: 271
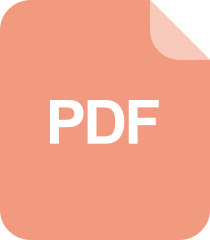
在地址栏输入192.168.1.1无法显示管理页面的解决方法
IPProcess1实现:
```java
import java.io.*;
import java.util.*;
public class IPProcess1 implements IPProcess {
@Override
public void process(File in, File out) throws IOException {
Map<String, Integer> ipMap = new HashMap<>();
BufferedReader reader = new BufferedReader(new FileReader(in));
String line;
while ((line = reader.readLine()) != null) {
ipMap.put(line, 1);
}
reader.close();
BufferedWriter writer = new BufferedWriter(new FileWriter(out));
for (String ip : ipMap.keySet()) {
writer.write(ip + "\n");
}
writer.close();
}
}
```
IPProcess1直接使用HashMap进行数据处理。将每行读入的IPv4地址作为key存入HashMap中,最后遍历HashMap输出所有出现过的IP地址。
IPProcess2实现:
```java
import java.io.*;
public class IPProcess2 implements IPProcess {
private static final int IP_LENGTH = 4; // IPv4地址长度
private static final int MAX_VALUE = 255; // IPv4每位最大值
private static final int MEMORY_SIZE = (int) Math.pow(MAX_VALUE + 1, IP_LENGTH); // 内存大小
@Override
public void process(File in, File out) throws IOException {
byte[] memory = new byte[MEMORY_SIZE]; // 创建byte数组,每一位表示一个IP地址
BufferedReader reader = new BufferedReader(new FileReader(in));
String line;
while ((line = reader.readLine()) != null) {
String[] parts = line.split("\\.");
int index = 0;
for (int i = 0; i < IP_LENGTH; i++) {
int value = Integer.parseInt(parts[i]);
index = index * (MAX_VALUE + 1) + value; // 根据IP地址计算在数组中的位置
}
memory[index] = 1; // 将对应位置的byte数组值置为1
}
reader.close();
BufferedWriter writer = new BufferedWriter(new FileWriter(out));
for (int i = 0; i < MEMORY_SIZE; i++) {
if (memory[i] == 1) { // 遍历byte数组,输出所有为1的位置对应的IP地址
int index = i;
int[] parts = new int[IP_LENGTH];
for (int j = IP_LENGTH - 1; j >= 0; j--) {
parts[j] = index % (MAX_VALUE + 1);
index /= (MAX_VALUE + 1);
}
writer.write(parts[0] + "." + parts[1] + "." + parts[2] + "." + parts[3] + "\n");
}
}
writer.close();
}
}
```
IPProcess2假设可用内存为1G,在内存中实现处理过程。创建byte数组,每一位表示一个IP地址,IPv4每位取值范围0-255,可采用位操作。读入每行IPv4地址后,根据IP地址计算在数组中的位置,将对应位置的byte数组值置为1。最后遍历byte数组,输出所有为1的位置对应的IP地址。
IPProcess3实现:
```java
import java.io.*;
public class IPProcess3 implements IPProcess {
private static final int IP_LENGTH = 4; // IPv4地址长度
private static final int MAX_VALUE = 255; // IPv4每位最大值
private static final int MEMORY_SIZE = (int) Math.pow(MAX_VALUE + 1, IP_LENGTH); // 内存大小
@Override
public void process(File in, File out) throws IOException {
RandomAccessFile inRAF = new RandomAccessFile(in, "r");
byte[] memory = new byte[MEMORY_SIZE]; // 创建byte数组,每一位表示一个IP地址
String line;
while ((line = inRAF.readLine()) != null) {
String[] parts = line.split("\\.");
int index = 0;
for (int i = 0; i < IP_LENGTH; i++) {
int value = Integer.parseInt(parts[i]);
index = index * (MAX_VALUE + 1) + value; // 根据IP地址计算在数组中的位置
}
memory[index] = 1; // 将对应位置的byte数组值置为1
}
inRAF.close();
RandomAccessFile outRAF = new RandomAccessFile(out, "rw");
for (int i = 0; i < MEMORY_SIZE; i++) {
if (memory[i] == 1) { // 遍历byte数组,输出所有为1的位置对应的IP地址
int index = i;
int[] parts = new int[IP_LENGTH];
for (int j = IP_LENGTH - 1; j >= 0; j--) {
parts[j] = index % (MAX_VALUE + 1);
index /= (MAX_VALUE + 1);
}
String ip = parts[0] + "." + parts[1] + "." + parts[2] + "." + parts[3] + "\n";
outRAF.write(ip.getBytes()); // 使用RandomAccessFile写入文件
}
}
outRAF.close();
}
}
```
IPProcess3假设内存非常少,必须在文件进行操作。使用RandomAccessFile读取输入文件,创建byte数组,每一位表示一个IP地址,IPv4每位取值范围0-255,可采用位操作。读入每行IPv4地址后,根据IP地址计算在数组中的位置,将对应位置的byte数组值置为1。最后遍历byte数组,输出所有为1的位置对应的IP地址,使用RandomAccessFile写入输出文件。
阅读全文
相关推荐
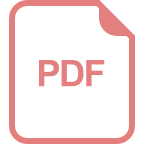
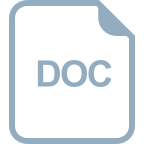

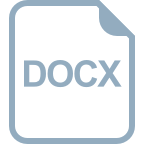
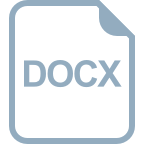
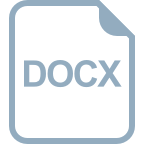
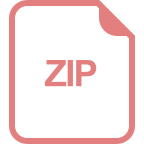
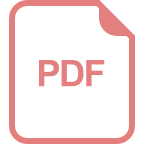
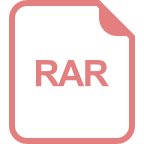
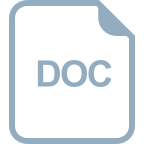







