java代码 输入解压密码并解压一个文件夹中所有zip文件
时间: 2024-03-03 11:53:59 浏览: 30
以下是Java代码,可以输入解压密码并解压一个文件夹中所有zip文件:
```java
import java.io.*;
import java.util.zip.ZipEntry;
import java.util.zip.ZipInputStream;
public class UnzipFolder {
public static void main(String[] args) {
String folderPath = "/path/to/folder"; // 文件夹路径
String password = "password"; // 解压密码
File folder = new File(folderPath);
if (folder.exists() && folder.isDirectory()) {
File[] files = folder.listFiles();
for (File file : files) {
if (file.getName().endsWith(".zip")) { // 如果是zip文件
unzip(file, password);
}
}
} else {
System.out.println("Folder does not exist or is not a directory.");
}
}
public static void unzip(File file, String password) {
byte[] buffer = new byte[1024];
try {
ZipInputStream zis = new ZipInputStream(new FileInputStream(file));
zis.setPassword(password.getBytes());
ZipEntry entry = zis.getNextEntry();
while (entry != null) {
String fileName = entry.getName();
File newFile = new File(file.getParentFile(), fileName);
if (entry.isDirectory()) {
newFile.mkdirs();
} else {
new File(newFile.getParent()).mkdirs();
FileOutputStream fos = new FileOutputStream(newFile);
int len;
while ((len = zis.read(buffer)) > 0) {
fos.write(buffer, 0, len);
}
fos.close();
}
entry = zis.getNextEntry();
}
zis.closeEntry();
zis.close();
System.out.println("Unzip " + file.getName() + " successfully.");
} catch (Exception e) {
System.out.println("Unzip " + file.getName() + " failed.");
e.printStackTrace();
}
}
}
```
在代码中,需要将`/path/to/folder`替换为实际的文件夹路径,将`password`替换为实际的解压密码。在调用`unzip`方法时,传入zip文件和解压密码即可解压文件。如果文件夹中有多个zip文件,程序会依次解压每个zip文件。
相关推荐
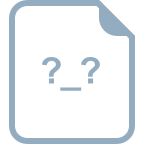














