相机回调函数中使用信号槽
时间: 2023-07-11 08:53:44 浏览: 53
在相机回调函数中使用信号槽可以实现回调函数和其他程序组件之间的通信。信号槽是一种 Qt 框架中用于实现事件驱动编程的机制,它可以让不同组件之间进行通信,比如在相机回调函数中获取到新的图像数据后,可以通过发射信号的方式通知其他组件进行图像处理或显示等操作。
为了在相机回调函数中使用信号槽,需要首先定义一个信号,比如 `newImage(QImage image)`,用于通知其他组件有新的图像数据到来;然后在相机回调函数中获取图像数据后,通过 `emit` 关键字发射该信号,将图像数据作为参数传递给槽函数;最后,在其他组件中定义一个槽函数,用于接收信号并处理图像数据。在这个过程中,需要确保信号和槽函数的参数类型和个数匹配,以保证数据能够正确传递。
相关问题
qt 相机回调函数中使用信号槽代码
以下是一个使用信号槽的 Qt 相机回调函数示例代码:
```c++
class Camera : public QObject
{
Q_OBJECT
public:
Camera(QObject *parent = nullptr)
: QObject(parent)
{
// 初始化相机
connect(&camera, SIGNAL(newImage(cv::Mat)), this, SLOT(onNewImage(cv::Mat)));
}
signals:
void newImage(QImage image);
private slots:
void onNewImage(cv::Mat image)
{
// 将 OpenCV Mat 转换为 QImage
QImage qImage(image.data, image.cols, image.rows, image.step, QImage::Format_RGB888);
// 发射信号,通知其他组件有新的图像数据到来
emit newImage(qImage);
}
private:
cv::VideoCapture camera;
};
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
Camera camera;
ImageProcessor imageProcessor;
ImageViewer imageViewer;
// 将相机信号连接到图像处理器的槽函数
QObject::connect(&camera, SIGNAL(newImage(QImage)), &imageProcessor, SLOT(processImage(QImage)));
// 将图像处理器信号连接到图像查看器的槽函数
QObject::connect(&imageProcessor, SIGNAL(processedImage(QImage)), &imageViewer, SLOT(showImage(QImage)));
// 开始捕获相机图像
camera.start();
return app.exec();
}
```
这个示例代码与前面的代码类似,不同之处在于它使用了 Qt 的信号槽机制。在 `Camera` 类的构造函数中,将 `newImage` 信号连接到 `onNewImage` 槽函数上,以便在获取到新的图像数据时发射该信号。在 `onNewImage` 槽函数中,将 OpenCV Mat 转换为 QImage,并通过 `emit` 关键字发射 `newImage` 信号,将图像数据传递给其他组件。
在 `main` 函数中,通过 `QObject::connect` 函数将 `Camera` 类的 `newImage` 信号连接到 `ImageProcessor` 类的 `processImage` 槽函数上,以便在图像数据到来时进行处理。然后,将 `ImageProcessor` 类的 `processedImage` 信号连接到 `ImageViewer` 类的 `showImage` 槽函数上,以便将处理后的图像显示出来。最后,在调用 `camera.start()` 函数启动相机捕获图像。
相机回调函数中使用信号槽实例代码
以下是一个使用信号槽的相机回调函数示例代码:
```c++
class Camera : public QObject
{
Q_OBJECT
public:
Camera(QObject *parent = nullptr)
: QObject(parent)
{
// 初始化相机
connect(&camera, &Camera::newImage, this, &Camera::onNewImage);
}
signals:
void newImage(QImage image);
private slots:
void onNewImage(cv::Mat image)
{
// 将 OpenCV Mat 转换为 QImage
QImage qImage(image.data, image.cols, image.rows, image.step, QImage::Format_RGB888);
// 发射信号,通知其他组件有新的图像数据到来
emit newImage(qImage);
}
private:
cv::VideoCapture camera;
};
int main(int argc, char *argv[])
{
QApplication app(argc, argv);
Camera camera;
ImageProcessor imageProcessor;
ImageViewer imageViewer;
// 将相机信号连接到图像处理器的槽函数
QObject::connect(&camera, &Camera::newImage, &imageProcessor, &ImageProcessor::processImage);
// 将图像处理器信号连接到图像查看器的槽函数
QObject::connect(&imageProcessor, &ImageProcessor::processedImage, &imageViewer, &ImageViewer::showImage);
// 开始捕获相机图像
camera.start();
return app.exec();
}
```
这个示例代码中,`Camera` 类是一个继承自 `QObject` 的相机类,它包含一个 `newImage` 信号,用于通知其他组件有新的图像数据到来。在 `Camera` 类的构造函数中,将 `newImage` 信号连接到 `onNewImage` 槽函数上,以便在获取到新的图像数据时发射该信号。在 `onNewImage` 槽函数中,将 OpenCV Mat 转换为 QImage,并通过 `emit` 关键字发射 `newImage` 信号,将图像数据传递给其他组件。
在 `main` 函数中,创建了一个 `Camera` 实例、一个 `ImageProcessor` 实例和一个 `ImageViewer` 实例,它们分别用于捕获相机图像、处理图像和显示图像。通过 `QObject::connect` 函数将 `Camera` 类的 `newImage` 信号连接到 `ImageProcessor` 类的 `processImage` 槽函数上,以便在图像数据到来时进行处理。然后,将 `ImageProcessor` 类的 `processedImage` 信号连接到 `ImageViewer` 类的 `showImage` 槽函数上,以便将处理后的图像显示出来。最后,在调用 `camera.start()` 函数启动相机捕获图像。
相关推荐
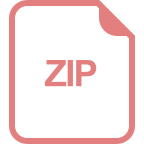
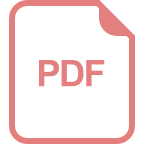












