一段使用STM32f103c8t6单片机开发板控制ws2812呼吸灯的代码
时间: 2023-11-11 22:02:52 浏览: 49
好的,以下是控制WS2812呼吸灯的STM32f103c8t6单片机开发板代码示例。请注意,此示例使用SysTick定时器来实现呼吸灯效果。
```c
#include "stm32f10x.h"
#define LED_NUM 1
void delay_us(uint32_t us) {
uint32_t count = us * (SystemCoreClock / 1000000);
while(count--);
}
void delay_ms(uint32_t ms) {
uint32_t count = ms * (SystemCoreClock / 1000);
while(count--);
}
void ws2812_set_color(uint8_t *buffer, uint8_t r, uint8_t g, uint8_t b) {
uint32_t temp = 0;
for (int i = 0; i < 8; i++) {
temp <<= 3;
if (g & 0x80) {
temp |= 0b110;
} else {
temp |= 0b100;
}
g <<= 1;
}
for (int i = 0; i < 8; i++) {
temp <<= 3;
if (r & 0x80) {
temp |= 0b110;
} else {
temp |= 0b100;
}
r <<= 1;
}
for (int i = 0; i < 8; i++) {
temp <<= 3;
if (b & 0x80) {
temp |= 0b110;
} else {
temp |= 0b100;
}
b <<= 1;
}
for (int i = 0; i < 24; i++) {
if (temp & 0x800000) {
buffer[i / 8] |= 0x80 >> i % 8;
}
temp <<= 1;
}
}
void ws2812_show(uint8_t *buffer) {
TIM_Cmd(TIM2, DISABLE);
for (int i = 0; i < LED_NUM * 24; i++) {
if (buffer[i / 8] & (0x80 >> i % 8)) {
GPIO_SetBits(GPIOA, GPIO_Pin_1);
delay_us(350);
GPIO_ResetBits(GPIOA, GPIO_Pin_1);
delay_us(900);
} else {
GPIO_SetBits(GPIOA, GPIO_Pin_1);
delay_us(900);
GPIO_ResetBits(GPIOA, GPIO_Pin_1);
delay_us(350);
}
}
TIM_Cmd(TIM2, ENABLE);
}
void pwm_init() {
GPIO_InitTypeDef GPIO_InitStructure;
TIM_TimeBaseInitTypeDef TIM_TimeBaseStructure;
TIM_OCInitTypeDef TIM_OCInitStructure;
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA | RCC_APB2Periph_AFIO, ENABLE);
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_0;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_AF_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
RCC_APB1PeriphClockCmd(RCC_APB1Periph_TIM2, ENABLE);
TIM_TimeBaseStructInit(&TIM_TimeBaseStructure);
TIM_TimeBaseStructure.TIM_Period = 71;
TIM_TimeBaseStructure.TIM_Prescaler = 0;
TIM_TimeBaseStructure.TIM_ClockDivision = 0;
TIM_TimeBaseStructure.TIM_CounterMode = TIM_CounterMode_Up;
TIM_TimeBaseInit(TIM2, &TIM_TimeBaseStructure);
TIM_OCInitStructInit(&TIM_OCInitStructure);
TIM_OCInitStructure.TIM_OCMode = TIM_OCMode_PWM1;
TIM_OCInitStructure.TIM_OutputState = TIM_OutputState_Enable;
TIM_OCInitStructure.TIM_Pulse = 35;
TIM_OCInitStructure.TIM_OCPolarity = TIM_OCPolarity_High;
TIM_OC2Init(TIM2, &TIM_OCInitStructure);
TIM_OC2PreloadConfig(TIM2, TIM_OCPreload_Enable);
TIM_Cmd(TIM2, ENABLE);
}
int main(void) {
pwm_init();
uint8_t buffer[LED_NUM * 24] = {0};
while (1) {
for (int i = 0; i <= 100; i++) {
uint8_t r = i;
uint8_t g = 100 - i;
uint8_t b = 0;
ws2812_set_color(buffer, r, g, b);
ws2812_show(buffer);
delay_ms(10);
}
for (int i = 100; i >= 0; i--) {
uint8_t r = i;
uint8_t g = 100 - i;
uint8_t b = 0;
ws2812_set_color(buffer, r, g, b);
ws2812_show(buffer);
delay_ms(10);
}
}
}
```
希望这个示例对你有所帮助!如果您有其他问题,请随时问我。
相关推荐
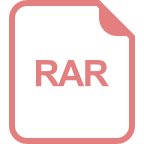
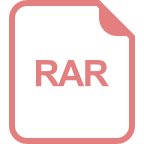
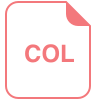
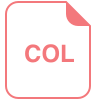
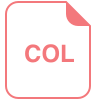
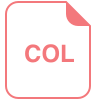
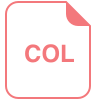










