html中使用js+table 实现分页
时间: 2023-11-15 08:04:18 浏览: 163
可以通过以下步骤使用 JavaScript 和 HTML 表格来实现分页:
1. 在 HTML 中创建一个表格,并设置 ID 和类名:
```
<table id="myTable" class="table">
<thead>
<tr>
<th>列1</th>
<th>列2</th>
<th>列3</th>
</tr>
</thead>
<tbody>
<tr>
<td>行1列1</td>
<td>行1列2</td>
<td>行1列3</td>
</tr>
<tr>
<td>行2列1</td>
<td>行2列2</td>
<td>行2列3</td>
</tr>
...
</tbody>
</table>
```
2. 在 JavaScript 中定义每页显示的行数和当前页码:
```
var rowsPerPage = 10; // 每页显示10行
var currentPage = 1; // 当前页码默认为1
```
3. 编写一个函数来显示当前页的行数:
```
function showTableRows() {
var table = document.getElementById('myTable');
var rows = table.getElementsByTagName('tr');
var startIndex = (currentPage - 1) * rowsPerPage + 1; // 计算当前页的起始行索引
var endIndex = startIndex + rowsPerPage - 1; // 计算当前页的结束行索引
for (var i = 0; i < rows.length; i++) {
if (i < startIndex || i > endIndex) {
rows[i].style.display = 'none'; // 隐藏不在当前页范围内的行
} else {
rows[i].style.display = ''; // 显示在当前页范围内的行
}
}
}
```
4. 编写一个函数来显示页码和添加事件监听器:
```
function showPagination() {
var table = document.getElementById('myTable');
var rows = table.getElementsByTagName('tr');
var totalPages = Math.ceil(rows.length / rowsPerPage); // 计算总页数
var pagination = document.createElement('div'); // 创建一个分页容器
pagination.className = 'pagination';
for (var i = 1; i <= totalPages; i++) {
var pageLink = document.createElement('a'); // 创建一个页码链接
pageLink.href = '#';
pageLink.innerHTML = i;
if (i === currentPage) {
pageLink.className = 'active'; // 当前页码高亮显示
}
pageLink.addEventListener('click', function(e) {
e.preventDefault();
currentPage = parseInt(this.innerHTML);
showTableRows();
showPagination();
});
pagination.appendChild(pageLink);
}
table.parentNode.insertBefore(pagination, table.nextSibling); // 将分页容器插入到表格后面
}
```
5. 调用上述两个函数来显示当前页和分页:
```
showTableRows();
showPagination();
```
6. 最后,添加一些 CSS 样式来美化分页:
```
.pagination {
margin-top: 10px;
}
.pagination a {
display: inline-block;
padding: 5px 10px;
margin-right: 5px;
border: 1px solid #ccc;
border-radius: 3px;
text-decoration: none;
}
.pagination a.active {
background-color: #007bff;
color: #fff;
border-color: #007bff;
}
```
以上就是使用 JavaScript 和 HTML 表格来实现分页的方法。
阅读全文
相关推荐
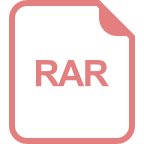
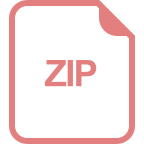
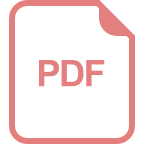
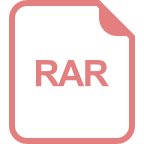
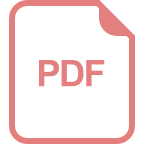
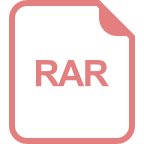
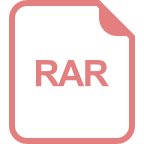
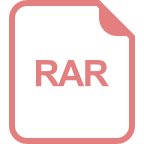
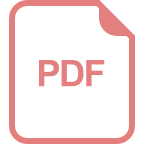
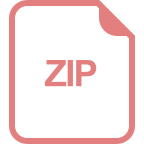
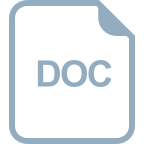



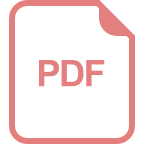