python中如何返回一张图片
时间: 2024-05-07 10:19:03 浏览: 99
在Python中,可以使用Pillow库来处理图片,并使用Flask框架来返回图片。
以下是一个示例代码:
```python
from flask import Flask, send_file
from PIL import Image
app = Flask(__name__)
@app.route('/get_image')
def get_image():
# 打开图片
img = Image.open('path/to/image.jpg')
# 将图片保存到内存中
img_io = io.BytesIO()
img.save(img_io, 'JPEG', quality=70)
# 返回图片给客户端
img_io.seek(0)
return send_file(img_io, mimetype='image/jpeg')
```
在这个示例中,当访问`/get_image`路径时,会返回路径为`path/to/image.jpg`的图片。首先,使用Pillow库打开图片,然后将图片保存到内存中。最后,使用Flask框架的`send_file`方法将图片返回给客户端。注意,这里的图片格式是JPEG格式,你也可以根据需要使用其他格式。
相关问题
python怎么输出一张图片
在Python中,你可以使用PIL(Python Imaging Library)或者其更现代的替代品Pillow库来输出图片。这里是一个简单的例子:
```python
from PIL import Image
# 加载图片
img = Image.open('image.jpg') # 如果图片位于同一目录下,直接写文件名;否则提供完整路径
# 创建一个新的图片文件
output_img = Image.new(img.mode, (new_width, new_height)) # 可选地调整尺寸
# 将原图粘贴到新图片上
output_img.paste(img, (x_offset, y_offset))
# 输出图片
output_img.save('output_image.jpg')
```
在这个例子中,你需要替换`image.jpg`为你要处理的图片文件名,`new_width`和`new_height`为新的宽度和高度(如果需要改变),以及`x_offset`和`y_offset`为原图在新图中的位置(默认值0表示居中)。
如果你想显示图片而不是保存,可以使用`display`模块,如`Image.show()`。
python函数调用返回图片
可以使用Python的Pillow库来创建和处理图像,然后将生成的图像保存到本地或在Web应用程序中返回给客户端。以下是一个示例函数,该函数使用Pillow库生成一张简单的红色图片,并将其返回给调用者:
```python
from PIL import Image
from io import BytesIO
def generate_image():
# Create a red image with size 200x200
img = Image.new('RGB', (200, 200), color = 'red')
# Save the image to a byte buffer
buffer = BytesIO()
img.save(buffer, 'JPEG')
buffer.seek(0)
# Return the byte buffer as a response
return buffer.getvalue()
```
这个函数会返回一张红色的200x200像素的JPEG图片,你可以将它保存到本地,或者在Web应用程序中返回给客户端。
阅读全文
相关推荐
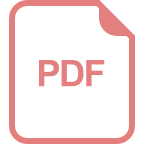












