找最大数 两种方法编写程序,创建包含10个数的数组,使用循环语句为数组赋值,输出其中最大值及对应的元素下标。考虑整型、小数、字符等不同类型数组的情况。方法1函数原型:intgetMaxIndex(inta[]intsize=10) int getMaxIndex(doublea[]intsize=10): int getMaxIndex(charaintsize=10); 方法2函数模板原型: template<typenameT> int getMaxIndex(Ta[]intsize=10);
时间: 2024-02-13 18:04:06 浏览: 57
方法1的实现:
```c++
#include <iostream>
using namespace std;
// 定义三个函数,分别处理整型、小数和字符数组
int getMaxIndex(int a[], int size = 10) {
int max = a[0];
int index = 0;
for (int i = 1; i < size; i++) {
if (a[i] > max) {
max = a[i];
index = i;
}
}
cout << "最大值是:" << max << ",对应的下标是:" << index << endl;
return index;
}
int getMaxIndex(double a[], int size = 10) {
double max = a[0];
int index = 0;
for (int i = 1; i < size; i++) {
if (a[i] > max) {
max = a[i];
index = i;
}
}
cout << "最大值是:" << max << ",对应的下标是:" << index << endl;
return index;
}
int getMaxIndex(char a[], int size = 10) {
char max = a[0];
int index = 0;
for (int i = 1; i < size; i++) {
if (a[i] > max) {
max = a[i];
index = i;
}
}
cout << "最大值是:" << max << ",对应的下标是:" << index << endl;
return index;
}
int main() {
int arrInt[] = {5, 9, 2, 1, 3, 6, 8, 7, 4, 0};
double arrDouble[] = {3.5, 1.2, 6.7, 2.3, 4.5, 9.8, 0.2, 8.1, 7.4, 5.6};
char arrChar[] = {'a', 'e', 'c', 'b', 'd', 'i', 'g', 'f', 'h', 'j'};
getMaxIndex(arrInt);
getMaxIndex(arrDouble);
getMaxIndex(arrChar);
return 0;
}
```
方法2的实现:
```c++
#include <iostream>
using namespace std;
template<typename T>
int getMaxIndex(T a[], int size = 10) {
T max = a[0];
int index = 0;
for (int i = 1; i < size; i++) {
if (a[i] > max) {
max = a[i];
index = i;
}
}
cout << "最大值是:" << max << ",对应的下标是:" << index << endl;
return index;
}
int main() {
int arrInt[] = {5, 9, 2, 1, 3, 6, 8, 7, 4, 0};
double arrDouble[] = {3.5, 1.2, 6.7, 2.3, 4.5, 9.8, 0.2, 8.1, 7.4, 5.6};
char arrChar[] = {'a', 'e', 'c', 'b', 'd', 'i', 'g', 'f', 'h', 'j'};
getMaxIndex(arrInt);
getMaxIndex(arrDouble);
getMaxIndex(arrChar);
return 0;
}
```
相关推荐
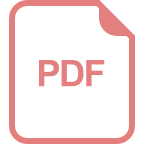
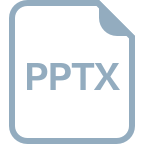
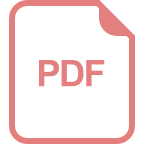














