python统计数组中的重复个数
时间: 2024-09-11 21:18:24 浏览: 93
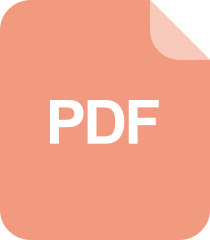
1、数组中重复的数字(python)
在Python中,如果你想要统计数组(列表)中的重复元素个数,可以使用几种不同的方法:
1. **使用collections模块的Counter**:
```python
from collections import Counter
arr = [1, 2, 3, 2, 4, 3, 5]
count_dict = Counter(arr)
duplicate_counts = {value: count for value, count in count_dict.items() if count > 1}
```
这将返回一个字典,其中键是重复的值,值是该值在列表中出现的次数。
2. **使用set数据结构**:
```python
arr = [1, 2, 3, 2, 4, 3, 5]
unique_elements = set(arr)
duplicate_counts = {element: arr.count(element) for element in unique_elements if arr.count(element) > 1}
```
这种方法先创建一个集合获取唯一元素,然后遍历计算每个元素的重复次数。
3. **使用numpy库** (如果数组很大):
```python
import numpy as np
arr = np.array([1, 2, 3, 2, 4, 3, 5])
counts = np.bincount(arr, minlength=max(arr) + 1)
duplicates = counts[counts > 1]
```
`np.bincount`函数能直接统计元素出现的次数,然后筛选出重复次数大于1的元素。
阅读全文
相关推荐
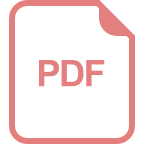
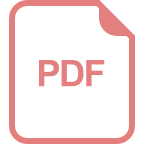
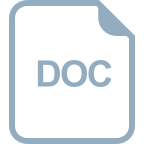













