子组件给父组件中的属性赋值
时间: 2023-11-03 15:00:38 浏览: 87
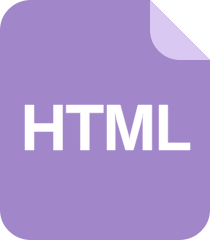
子组件向父组件传值.html
在React中,子组件不能直接修改父组件的属性。但是可以通过props将父组件中的方法传递给子组件,然后在子组件中调用该方法来修改父组件的属性。
例如,假设父组件中有一个属性count,并且有一个方法handleCountChange,可以通过以下方式将该方法传递给子组件:
```jsx
class ParentComponent extends React.Component {
constructor(props) {
super(props);
this.state = {
count: 0
};
this.handleCountChange = this.handleCountChange.bind(this);
}
handleCountChange(newCount) {
this.setState({ count: newCount });
}
render() {
return (
<div>
<ChildComponent
count={this.state.count}
onCountChange={this.handleCountChange}
/>
</div>
);
}
}
```
在子组件中,可以通过调用onCountChange方法来修改父组件中的count属性:
```jsx
class ChildComponent extends React.Component {
constructor(props) {
super(props);
this.handleButtonClick = this.handleButtonClick.bind(this);
}
handleButtonClick() {
const newCount = this.props.count + 1;
this.props.onCountChange(newCount);
}
render() {
return (
<div>
<button onClick={this.handleButtonClick}>Increase Count</button>
</div>
);
}
}
```
在上面的代码中,当子组件中的按钮被点击时,会调用handleButtonClick方法,该方法会将父组件中的count属性加1,并通过onCountChange方法将新的count值传递给父组件,从而实现修改父组件属性的目的。
阅读全文
相关推荐
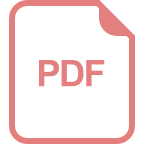

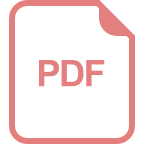
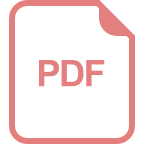












