linux 下C++程序std::thread多线程如何减少CPU占用率,请分别举例说明
时间: 2024-04-18 12:33:49 浏览: 21
当使用C++程序中的std::thread创建多线程时,可以采取以下方法来降低CPU占用率:
1. 调整线程的睡眠时间:
```cpp
#include <iostream>
#include <thread>
#include <chrono>
void threadFunc() {
while (true) {
// 在线程函数中加入适当的睡眠时间,降低CPU占用率
std::this_thread::sleep_for(std::chrono::milliseconds(10));
// 其他操作
}
}
int main() {
std::thread t(threadFunc);
t.join();
return 0;
}
```
在上述示例中,线程函数`threadFunc`在每次循环时都会睡眠10毫秒,从而降低了CPU占用率。根据实际需求,可以调整睡眠时间。
2. 使用互斥锁和条件变量:
```cpp
#include <iostream>
#include <thread>
#include <mutex>
#include <condition_variable>
std::mutex mtx;
std::condition_variable cv;
bool isReady = false;
void threadFunc() {
std::unique_lock<std::mutex> lock(mtx);
while (true) {
// 等待条件变量
cv.wait(lock, []{ return isReady; });
// 其他操作
}
}
int main() {
std::thread t(threadFunc);
// 模拟主线程设置条件变量的状态
std::this_thread::sleep_for(std::chrono::seconds(1));
{
std::lock_guard<std::mutex> lock(mtx);
isReady = true;
}
cv.notify_one();
t.join();
return 0;
}
```
在上述示例中,线程函数`threadFunc`会等待条件变量`isReady`为true时才执行其他操作。通过使用互斥锁和条件变量,可以避免线程不必要地竞争资源,从而降低CPU占用率。
这些是常见的方法,具体的应用场景可能需要根据实际需求进行调整和扩展。同时,还可以考虑使用线程池等更高级的技术来管理多线程,以进一步减少CPU占用率。
相关推荐
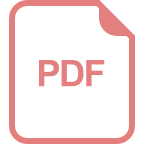














