定义一个主类,在主类的main方法中测试上述 Student类, 即 使用Student类的构造方法创建对象,然后调用其成员方法。
时间: 2024-09-25 09:17:30 浏览: 52
在Java中,首先你需要定义一个主类(例如叫做MainClass),并在其中编写一个`main`方法来测试`Student`类。假设`Student`类已经定义好了,包含姓名(name)、年龄(age)和成绩(score)等属性,以及获取信息(displayInfo)和计算平均分(calculateAverageScore)的方法。下面是主类的示例:
```java
public class MainClass {
public static void main(String[] args) {
// 创建 Student 类的对象
Student student = new Student("张三", 18, 90); // 使用构造方法初始化学生
// 调用成员方法
student.displayInfo(); // 展示学生信息
double averageScore = student.calculateAverageScore(); // 计算平均分并打印结果
// 示例:如果有多个成绩,可以像下面这样更新并再次计算平均分
student.addScore(95);
student.addScore(88);
System.out.println("添加成绩后的平均分:" + student.calculateAverageScore());
}
}
class Student {
private String name;
private int age;
private List<Integer> scores = new ArrayList<>();
// 构造方法
public Student(String name, int age, int score) {
this.name = name;
this.age = age;
scores.add(score);
}
// 成员方法
public void displayInfo() {
System.out.println("姓名:" + name + ", 年龄:" + age);
}
// 添加成绩
public void addScore(int score) {
scores.add(score);
}
// 计算平均分
public double calculateAverageScore() {
if (scores.isEmpty()) {
return 0;
}
double sum = scores.stream().mapToDouble(Integer::intValue).sum();
return sum / scores.size();
}
}
```
在这个例子中,我们在`main`方法里创建了一个`Student`对象,并调用了它的成员方法展示信息和计算平均分。如果需要增加更多的分数,我们可以直接操作`scores`列表并再次计算。
阅读全文
相关推荐
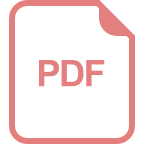
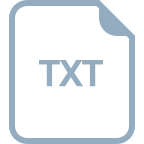
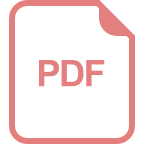












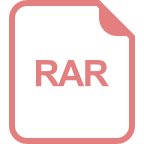
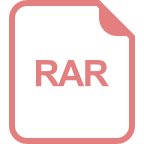