采用顺序表示的储存方式,用C语言实现线性表的创建、插入、合并算法
时间: 2024-09-23 22:07:35 浏览: 36
在C语言中,我们可以使用数组或动态内存分配(如`malloc()`)来实现基于顺序存储的线性表。这里我将分别介绍如何创建、插入和合并线性表。
**1. 创建线性表**
如果你选择使用数组,创建一个固定大小的线性表可以像这样:
```c
#define MAX_SIZE 10 // 定义最大元素数量
int linear_list[MAX_SIZE]; // 数组用于线性表
// 初始化线性表为空
void create_linear_list() {
for (int i = 0; i < MAX_SIZE; i++) {
linear_list[i] = 0; // 或者设置为初始值
}
}
```
如果需要动态扩展,你可以使用`malloc`:
```c
#include <stdlib.h>
typedef struct Node {
int data;
struct Node* next;
} Node;
Node* create_dynamic_list() {
Node* list = malloc(sizeof(Node)); // 分配内存
list->data = 0;
list->next = NULL;
return list;
}
```
**2. 插入操作**
- **在数组中插入**:
如果你知道要插入的位置,可以通过移动其他元素来插入:
```c
void insert_in_array(int index, int value) {
if (index < 0 || index >= MAX_SIZE) {
printf("Invalid index.\n");
return;
}
for (int i = MAX_SIZE - 1; i >= index; i--) {
linear_list[i + 1] = linear_list[i];
}
linear_list[index] = value;
}
// 动态数组插入类似,需考虑扩展容量的情况
```
- **链式表中插入**:
在链表中,可以在节点之间插入新节点:
```c
void insert_in_linked_list(Node** head, int value, int position) {
Node* newNode = malloc(sizeof(Node));
newNode->data = value;
newNode->next = *head;
if (position == 0) { // 插入到头部
*head = newNode;
} else {
Node* temp = *head;
for (int i = 0; i < position - 1 && temp != NULL; i++) {
temp = temp->next;
}
if (temp != NULL) {
newNode->next = temp->next;
temp->next = newNode;
} else {
printf("Invalid position.\n");
}
}
}
```
**3. 合并两个线性表**
假设你有两个已排序的线性表(比如两个数组),可以先比较它们的第一个元素,然后将较小的那个添加到结果数组中,重复这个过程直到一个列表为空:
```c
void merge_sorted_arrays(int arr1[], int arr2[], int len1, int len2, int result[]) {
int i = 0, j = 0, k = 0;
while (i < len1 && j < len2) {
if (arr1[i] <= arr2[j]) {
result[k++] = arr1[i++];
} else {
result[k++] = arr2[j++];
}
}
// 处理剩余元素(如果有的话)
while (i < len1) {
result[k++] = arr1[i++];
}
while (j < len2) {
result[k++] = arr2[j++];
}
}
```
阅读全文
相关推荐
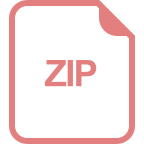
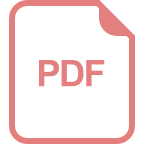
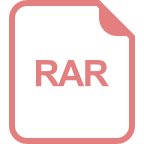
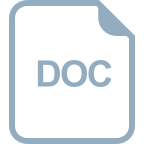
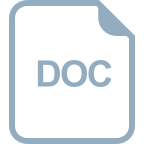
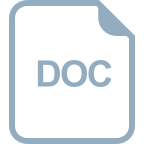
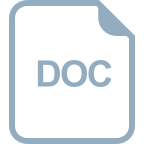
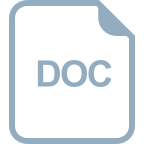
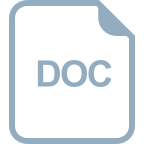
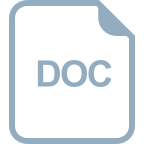
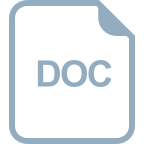
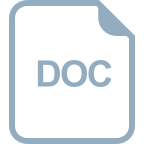
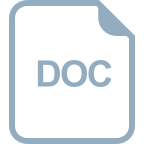


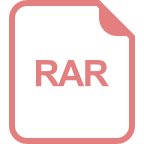
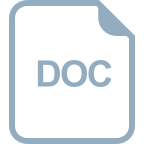
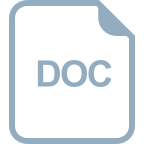
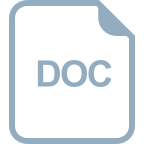