C语言中输出所有水仙花数
时间: 2024-11-06 14:20:40 浏览: 27
在C语言中,为了输出所有的水仙花数,我们可以编写一个循环遍历从最小的三位数到最大的三位数的程序,并对每个数进行检查。这里有一个简单的示例:
```c
#include <stdio.h>
void print_pythagorean_triples() {
for (int num = 100; num <= 999; ++num) { // 遍历三位数
int hundreds = num / 100;
int tens = (num % 100) / 10;
int units = num % 10;
// 如果每一位的立方和等于原数,则打印出来
if (hundreds * hundreds * hundreds + tens * tens * tens + units * units * units == num) {
printf("%d 是一个水仙花数。\n", num);
}
}
}
int main() {
print_pythagorean_triples();
return 0;
}
```
这段代码会依次检查每个三位数,如果发现符合条件(即各个位数的立方和等于该数本身),就将其打印出来。
相关问题
编写一个c语言程序输出所有水仙花数
好的,以下是C语言程序输出所有水仙花数的代码:
```
#include <stdio.h>
int main() {
int i, j, k, n;
printf("水仙花数有:\n");
for (n = 100; n <= 999; n++) {
i = n / 100;
j = n / 10 % 10;
k = n % 10;
if (n == i * i * i + j * j * j + k * k * k) {
printf("%d\n", n);
}
}
return 0;
}
```
运行之后,程序会输出所有水仙花数。如果您有其他的问题,欢迎随时问我。
c语言输出所有的水仙花数
以下是C语言输出所有水仙花数的代码和解释:
```c
#include <stdio.h>
int main() {
// 从100开始进行判断,到999结束
for (int num = 100; num < 1000; num++) {
// 数学方法获取个十百位上的数字
int f = num / 100;
int s = (num - f * 100) / 10 ;
int t = num % 10;
// 判断是否为水仙花数
if (num == f * f * f + s * s * s + t * t * t) {
// 输出水仙花数
printf("水仙花数为 %d \n", num);
}
}
return 0;
}
```
解释:
1. 首先,我们从100开始进行判断,到999结束,因为水仙花数是三位数。
2. 然后,我们使用数学方法获取个十百位上的数字,分别用f、s、t表示。
3. 接着,我们判断是否为水仙花数,如果是,就输出该数。
4. 最后,我们将所有的水仙花数输出。
阅读全文
相关推荐
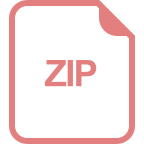
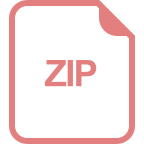













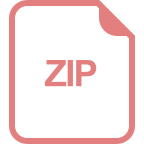
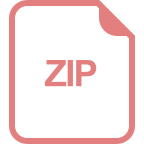