a. 要有菜单,并能循环使用: 设计一个交互式菜单,用户可以通过菜单选择计算器的不同功能。菜单应当能够循环使用,直到用户选择退出。 b. 用分支结构实现基本数据的运算: 使用if-else或switch-case等分支结构实现加、减、乘、除等基本数据运算,用C++写
时间: 2024-12-12 17:20:24 浏览: 13
a. 要设计一个交互式菜单并能循环使用的计算器程序,你可以按照以下步骤操作:
```cpp
#include <iostream>
using namespace std;
enum Operation {
ADD,
SUBTRACT,
MULTIPLY,
DIVIDE,
EXIT
};
void printMenu() {
cout << "请选择操作:\n";
cout << "1. 加法\n";
cout << "2. 减法\n";
cout << "3. 乘法\n";
cout << "4. 除法\n";
cout << "5. 退出\n";
}
int getMenuChoice() {
int choice;
do {
cout << "请输入您的选择(1-5): ";
cin >> choice;
} while (choice < 1 || choice > 5);
return choice;
}
double performOperation(double num1, double num2, Operation op) {
switch (op) {
case ADD:
return num1 + num2;
case SUBTRACT:
return num1 - num2;
case MULTIPLY:
return num1 * num2;
case DIVIDE:
if (num2 != 0)
return num1 / num2;
else
throw runtime_error("除数不能为零");
default:
break;
}
return 0; // 防止未处理的情况,默认返回0
}
int main() {
double num1, num2;
Operation currentOp = EXIT;
while (currentOp != EXIT) {
try {
printMenu();
currentOp = (Operation)getMenuChoice();
if (currentOp != EXIT) {
cout << "请输入两个数字: ";
cin >> num1 >> num2;
double result = performOperation(num1, num2, currentOp);
cout << "结果: " << result << endl;
}
} catch (runtime_error &e) {
cerr << e.what() << endl;
}
}
cout << "感谢使用计算器,再见!\n";
return 0;
}
```
在这个程序中,我们首先定义了菜单选项和对应的运算符枚举,然后创建了一个打印菜单、获取用户输入以及执行相应运算的函数。在主循环中,只要用户没有选择退出,就会继续接收输入并计算结果。
b. 分支结构部分已经包含在上述代码中,就是通过`switch-case`结构实现了加、减、乘、除的操作。
阅读全文
相关推荐
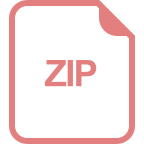
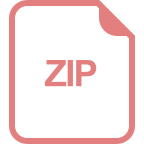
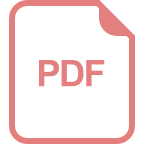















