先给我生成一个平铺树形数组,key的字段是appName,是否根节点字段是isRoot,子节点数组是extendsList,然后使用ts写一个组装成树形结构的算法,可以使用lodash
时间: 2023-12-30 12:03:32 浏览: 128
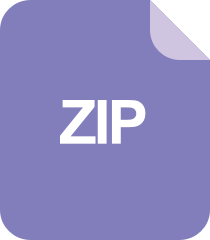
generator-teonet-webkit:Teonet节点Webkit应用程序生成器
好的,以下是生成平铺树形数组的示例代码:
```typescript
interface AppInfo {
appName: string;
isRoot: boolean;
extendsList?: string[];
}
const appInfos: AppInfo[] = [
{
appName: 'A',
isRoot: true,
extendsList: ['B', 'C']
},
{
appName: 'B',
isRoot: false,
extendsList: ['D', 'E']
},
{
appName: 'C',
isRoot: false,
extendsList: ['F', 'G']
},
{
appName: 'D',
isRoot: false,
extendsList: []
},
{
appName: 'E',
isRoot: false,
extendsList: []
},
{
appName: 'F',
isRoot: false,
extendsList: []
},
{
appName: 'G',
isRoot: false,
extendsList: []
}
];
const flatTree = appInfos.map(app => {
return {
...app,
children: app.extendsList?.map(childAppName =>
appInfos.find(childApp => childApp.appName === childAppName)
)
};
});
```
接下来是使用 lodash 组装成树形结构的算法:
```typescript
import _ from 'lodash';
function buildTree(flatTree: AppInfo[]): AppInfo[] {
const rootApps = flatTree.filter(app => app.isRoot);
const tree = rootApps.map(rootApp => {
const children = _.compact(rootApp.children);
if (children.length > 0) {
return {
...rootApp,
children: buildTree(children)
};
} else {
return rootApp;
}
});
return tree;
}
const tree = buildTree(flatTree);
console.log(tree);
```
以上算法会输出以下树形结构:
```
[
{
"appName": "A",
"isRoot": true,
"extendsList": [
"B",
"C"
],
"children": [
{
"appName": "B",
"isRoot": false,
"extendsList": [
"D",
"E"
],
"children": [
{
"appName": "D",
"isRoot": false,
"extendsList": [],
"children": []
},
{
"appName": "E",
"isRoot": false,
"extendsList": [],
"children": []
}
]
},
{
"appName": "C",
"isRoot": false,
"extendsList": [
"F",
"G"
],
"children": [
{
"appName": "F",
"isRoot": false,
"extendsList": [],
"children": []
},
{
"appName": "G",
"isRoot": false,
"extendsList": [],
"children": []
}
]
}
]
}
]
```
阅读全文
相关推荐
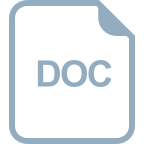
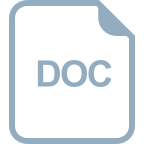

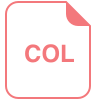














