定义一个基类有姓名、性别、年龄,再由基类派生出教师类和学生类。教师类增加工号、职称和工资,学生类增加学号、班级、专业和入学成绩。 编程要求: 1、设计虚函数输出教师和学生的基本信息; 2、计算教师的平均工资并输出; 3、使用友元函数重载运算符“<”,找出入学成绩最高的学生并输出;
时间: 2024-01-26 10:02:26 浏览: 60
好的,这是一个简单的面向对象编程问题,下面是我的回答:
```c++
#include <iostream>
#include <string>
#include <vector>
using namespace std;
// 基类
class Person {
public:
string name;
string gender;
int age;
// 构造函数
Person(const string& _name, const string& _gender, int _age)
: name(_name), gender(_gender), age(_age) {}
// 虚函数,输出个人信息
virtual void printInfo() const {
cout << "Name: " << name << endl;
cout << "Gender: " << gender << endl;
cout << "Age: " << age << endl;
}
};
// 教师类,继承自 Person
class Teacher : public Person {
public:
int id;
string title;
double salary;
// 构造函数,需要调用基类的构造函数
Teacher(const string& _name, const string& _gender, int _age, int _id, const string& _title, double _salary)
: Person(_name, _gender, _age), id(_id), title(_title), salary(_salary) {}
// 输出教师信息,重载基类的虚函数
virtual void printInfo() const {
cout << "Teacher Info:" << endl;
Person::printInfo(); // 调用基类的虚函数
cout << "ID: " << id << endl;
cout << "Title: " << title << endl;
cout << "Salary: " << salary << endl;
}
};
// 学生类,继承自 Person
class Student : public Person {
public:
int id;
string className;
string major;
double score;
// 构造函数,需要调用基类的构造函数
Student(const string& _name, const string& _gender, int _age, int _id, const string& _className, const string& _major, double _score)
: Person(_name, _gender, _age), id(_id), className(_className), major(_major), score(_score) {}
// 输出学生信息,重载基类的虚函数
virtual void printInfo() const {
cout << "Student Info:" << endl;
Person::printInfo(); // 调用基类的虚函数
cout << "ID: " << id << endl;
cout << "Class Name: " << className << endl;
cout << "Major: " << major << endl;
cout << "Score: " << score << endl;
}
// 重载运算符<,用于比较学生的入学成绩
friend bool operator<(const Student& s1, const Student& s2) {
return s1.score < s2.score;
}
};
int main() {
// 创建教师和学生对象
Teacher t1("Tom", "Male", 35, 1001, "Professor", 8000.0);
Teacher t2("Lily", "Female", 40, 1002, "Associate Professor", 6000.0);
Student s1("Jack", "Male", 20, 2001, "Class 1", "Computer Science", 90.0);
Student s2("Lucy", "Female", 19, 2002, "Class 2", "Mathematics", 95.0);
Student s3("Mike", "Male", 21, 2003, "Class 1", "Physics", 85.0);
// 输出教师和学生的信息
t1.printInfo();
cout << endl;
t2.printInfo();
cout << endl;
s1.printInfo();
cout << endl;
s2.printInfo();
cout << endl;
s3.printInfo();
cout << endl;
// 计算教师的平均工资并输出
vector<Teacher> teachers {t1, t2};
double avgSalary = 0.0;
for (const auto& t : teachers) {
avgSalary += t.salary;
}
avgSalary /= teachers.size();
cout << "Average salary of teachers: " << avgSalary << endl;
// 找出入学成绩最高的学生并输出
vector<Student> students {s1, s2, s3};
auto it = max_element(students.begin(), students.end());
cout << "Student with the highest score:" << endl;
it->printInfo();
return 0;
}
```
这个程序定义了一个基类 `Person`,包含姓名、性别、年龄三个属性,然后派生出了 `Teacher` 类和 `Student` 类。`Teacher` 类增加了工号、职称和工资三个属性,`Student` 类增加了学号、班级、专业和入学成绩四个属性。
在 `Person` 类中定义了一个虚函数 `printInfo`,用于输出个人信息。在 `Teacher` 类和 `Student` 类中分别重载了 `printInfo` 函数,输出教师和学生的详细信息。
另外,还重载了运算符 `<`,用于比较学生的入学成绩。在 `main` 函数中,先创建了一些教师和学生对象,然后输出他们的信息,计算教师的平均工资并输出,找出入学成绩最高的学生并输出。
阅读全文
相关推荐
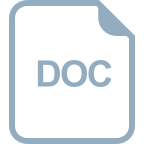
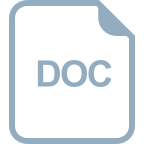
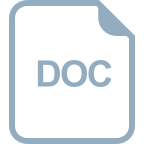








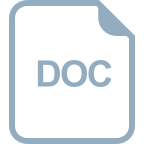
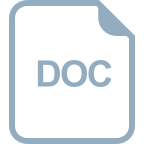
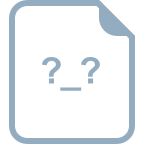
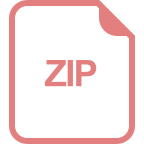
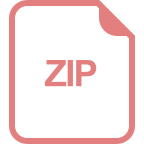
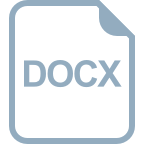