springboot异步任务
时间: 2023-06-29 16:06:26 浏览: 129
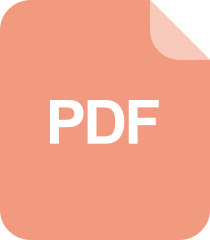
Springboot中异步
在 Spring Boot 中,异步任务可以使用 `@Async` 注解来实现。使用 `@Async` 注解的方法会在一个单独的线程中执行,从而避免了长时间的阻塞。
以下是实现异步任务的步骤:
1. 在 Spring Boot 应用的主类上添加 `@EnableAsync` 注解,启用异步任务。
```java
@EnableAsync
@SpringBootApplication
public class Application {
// ...
}
```
2. 在需要异步执行的方法上添加 `@Async` 注解。该方法的返回值类型必须是 `java.util.concurrent.Future`,以便在需要时获取异步任务的执行结果。
```java
@Service
public class MyService {
@Async
public Future<String> doSomething() throws InterruptedException {
// 模拟一个耗时的操作
Thread.sleep(5000);
return new AsyncResult<>("Done");
}
}
```
3. 在需要调用异步方法的地方,使用 `Future` 对象获取异步任务的执行结果。
```java
@RestController
public class MyController {
@Autowired
private MyService myService;
@GetMapping("/async")
public String asyncMethod() throws InterruptedException, ExecutionException {
Future<String> future = myService.doSomething();
while (!future.isDone()) {
// 等待异步任务完成
Thread.sleep(1000);
}
return future.get();
}
}
```
以上就是使用 `@Async` 注解实现异步任务的步骤。需要注意的是,异步任务的执行需要在一个单独的线程中进行,因此需要在应用程序中配置线程池,以便管理异步任务的线程。可以使用 Spring Boot 中的 `ThreadPoolTaskExecutor` 来实现线程池的配置。
阅读全文
相关推荐


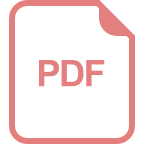
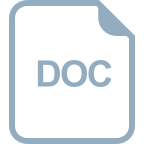



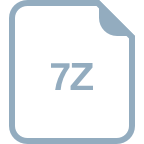







