用c++语言实现
时间: 2024-02-28 11:53:54 浏览: 107
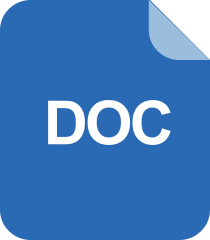
c++代码的实现
好的,以下是用 C++ 实现的代码:
```cpp
#include <iostream>
using namespace std;
struct Node {
int id;
int score;
Node *next;
};
Node* merge(Node *head_a, Node *head_b) {
Node *head = NULL;
Node *tail = NULL;
while (head_a != NULL && head_b != NULL) {
Node *node;
if (head_a->id < head_b->id) {
node = head_a;
head_a = head_a->next;
} else {
node = head_b;
head_b = head_b->next;
}
if (head == NULL) {
head = node;
} else {
tail->next = node;
}
tail = node;
}
if (head_a != NULL) {
if (head == NULL) {
head = head_a;
} else {
tail->next = head_a;
}
}
if (head_b != NULL) {
if (head == NULL) {
head = head_b;
} else {
tail->next = head_b;
}
}
return head;
}
int main() {
int n, m;
cin >> n >> m;
Node *head_a = NULL;
Node *tail_a = NULL;
for (int i = 0; i < n; i++) {
int id, score;
cin >> id >> score;
Node *node = new Node;
node->id = id;
node->score = score;
node->next = NULL;
if (tail_a == NULL) {
head_a = node;
} else {
tail_a->next = node;
}
tail_a = node;
}
Node *head_b = NULL;
Node *tail_b = NULL;
for (int i = 0; i < m; i++) {
int id, score;
cin >> id >> score;
Node *node = new Node;
node->id = id;
node->score = score;
node->next = NULL;
if (tail_b == NULL) {
head_b = node;
} else {
tail_b->next = node;
}
tail_b = node;
}
Node *head = merge(head_a, head_b);
Node *node = head;
while (node != NULL) {
cout << node->id << " " << node->score << endl;
node = node->next;
}
return 0;
}
```
思路和 Python 版本的代码基本一致,只是用了 C++ 的语法和动态内存分配来管理链表结点的内存。
具体来说,定义了一个 `Node` 结构体表示链表结点,包含学号、成绩和指向下一个结点的指针。然后用 `merge` 函数将两个链表合并,返回合并后的新链表的头指针。在合并的过程中,使用两个指针分别指向 a 和 b 两个链表的头结点,比较它们的学号大小,将较小的结点加入新链表中,然后移动对应链表的指针。最后可能会有一些结点没有加入新链表中,需要将它们依次加入到新链表的尾部。
在主函数中,读入输入数据,构建出 a 和 b 两个链表的结点,然后调用 `merge` 函数将两个链表合并得到新的链表头指针,最后按顺序输出新链表的每个结点的学号和成绩属性即可。注意释放动态分配的结点内存。
阅读全文
相关推荐
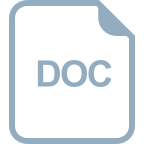
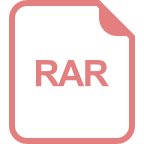
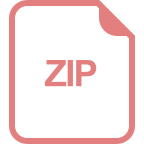
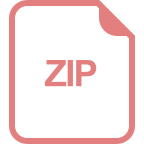
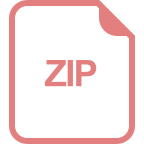
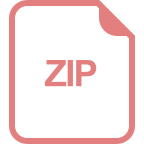
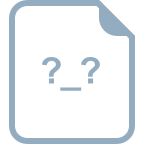