selenium iframe嵌套切换
时间: 2023-11-03 11:05:13 浏览: 66
可以使用 switch_to.frame() 方法来切换嵌套的 iframe。首先需要先定位到外层的 iframe,然后再切换到内层的 iframe。例如:
```python
from selenium import webdriver
driver = webdriver.Chrome()
# 访问网页
driver.get("http://www.example.com")
# 定位外层的 iframe
outer_iframe = driver.find_element_by_css_selector("#outer-iframe")
# 切换到外层的 iframe
driver.switch_to.frame(outer_iframe)
# 定位内层的 iframe
inner_iframe = driver.find_element_by_css_selector("#inner-iframe")
# 切换到内层的 iframe
driver.switch_to.frame(inner_iframe)
# 在内层的 iframe 中进行操作...
# 切换回外层的 iframe
driver.switch_to.parent_frame()
# 切换回主文档
driver.switch_to.default_content()
# 关闭浏览器
driver.quit()
```
相关问题
selenium用iframe切换框架
使用selenium切换iframe框架,可以按照以下步骤进行:
1. 使用driver.switch_to.frame()方法进入iframe框架,其中参数可以是iframe的id、name或者WebElement对象。
2. 在iframe框架中进行操作,比如查找元素、点击按钮等。
3. 使用driver.switch_to.default_content()方法退出iframe框架,返回到主页面。
需要注意的是,如果iframe框架嵌套多层,则需要多次使用switch_to.frame()方法进入对应的框架。
selenium 怎么跳入嵌套iframe
如果要在Selenium中跳转到嵌套的iframe中,需要使用`switch_to.frame()`方法。该方法可以接收iframe的索引、iframe的name或iframe元素作为参数。
以下是使用索引的示例:
```
driver.switch_to.frame(0)
```
以上代码将跳转到页面中第一个iframe。
以下是使用name的示例:
```
driver.switch_to.frame("myFrame")
```
以上代码将跳转到name为“myFrame”的iframe。
以下是使用iframe元素的示例:
```
iframe = driver.find_element_by_xpath("//iframe[@id='myFrame']")
driver.switch_to.frame(iframe)
```
以上代码将跳转到id为“myFrame”的iframe。
注意:当你完成在iframe中的操作后,需要使用`switch_to.default_content()`方法切换回主窗口。
相关推荐
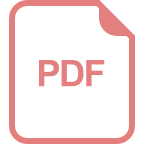













